MVVM (Model-View-ViewModel) is a design pattern used in software engineering that helps to separate the user interface logic from the business logic. In iOS development, MVVM is used with SwiftUI to build scalable and testable applications. In this blog, we will learn how to use MVVM in SwiftUI by building a simple app that fetches data from a reachable API.
The Model-View-ViewModel (MVVM) Design Pattern
The MVVM design pattern separates the user interface (View) from the business logic (ViewModel) and the data (Model). It works by having the View display data from the ViewModel, which in turn gets the data from the Model. The ViewModel acts as a mediator between the View and the Model. The View is responsible for displaying the data, the ViewModel is responsible for managing the data and the Model is responsible for providing the data.
In MVVM, the View and ViewModel are decoupled from each other, which means that changes in the ViewModel do not affect the View directly. Instead, the ViewModel uses the Observer pattern to notify the View of any changes in the data.
Building a Simple SwiftUI App using MVVM
Let’s build a simple SwiftUI app that fetches data from a reachable API and displays it in a list using the MVVM design pattern.
Step 1: Create a new SwiftUI Project
First, create a new SwiftUI project in Xcode by selecting “File” > “New” > “Project” and then choosing “App” under the iOS section. Give your project a name and click “Create”.
Step 2: Create the Model
The Model is responsible for providing the data to the ViewModel. In this app, we will fetch data from a reachable API and parse it using a Codable data model.
Create a new Swift file called “Post.swift” and add the following code:
struct Post: Codable {
let id: Int
let title: String
let body: String
}
Step 3: Create the ViewModel
The ViewModel is responsible for managing the data and providing it to the View. In this app, we will fetch data from the API using the URLSession and decode it using the Codable data model.
Create a new Swift file called “PostViewModel.swift” and add the following code:
import SwiftUI
class PostViewModel: ObservableObject {
@Published var posts = [Post]()
init() {
fetchPosts()
}
func fetchPosts() {
guard let url = URL(string: "https://jsonplaceholder.typicode.com/posts") else {
return
}
URLSession.shared.dataTask(with: url) { data, response, error in
guard let data = data else {
return
}
do {
let posts = try JSONDecoder().decode([Post].self, from: data)
DispatchQueue.main.async {
self.posts = posts
}
} catch {
print(error)
}
}.resume()
}
}
Step 4: Create the View
The View is responsible for displaying the data provided by the ViewModel. In this app, we will display the data in a list.
Open the ContentView.swift file and add the following code:
import SwiftUI
struct ContentView: View {
@ObservedObject var viewModel = PostViewModel()
var body: some View {
List(viewModel.posts, id: \.id) { post in
Section(header: Text("Book Title"), footer: Text("End of List")) {
VStack(alignment: .leading) {
Text(post.title)
.font(.headline)
Text(post.body)
.font(.subheadline)
.foregroundColor(.gray)
}
}
}
}
}
Step 5: Run the App
Build and run the app in Xcode using the simulator or a physical device. The app should fetch data from the API and display it in a list.
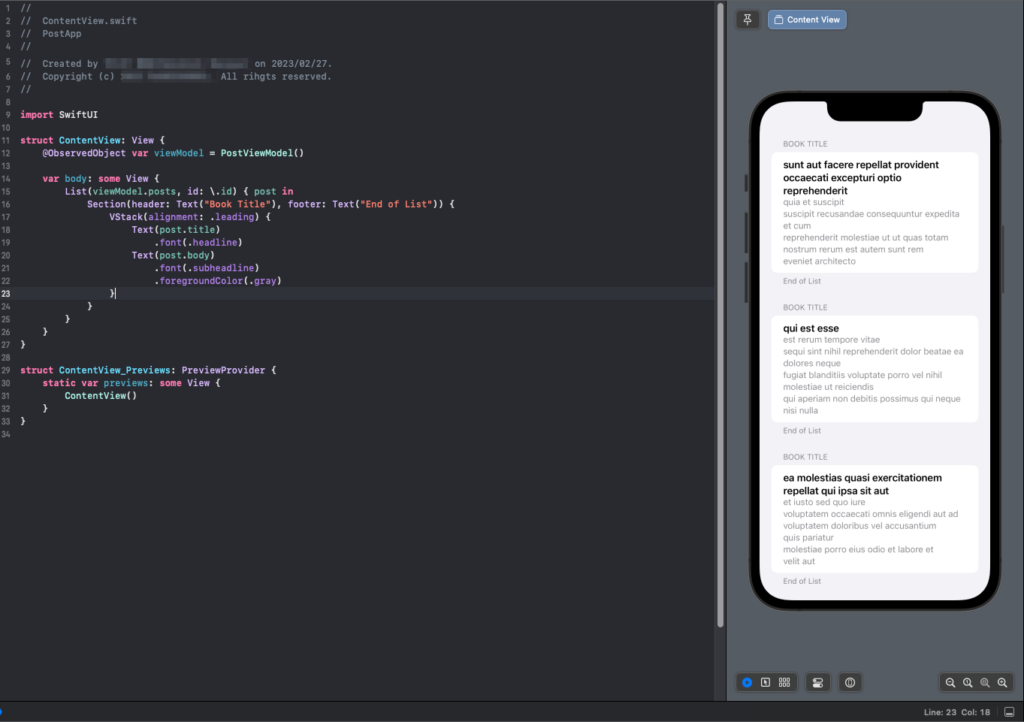
Conclusion
In this blog, we learned how to use the MVVM design pattern with SwiftUI to build a simple app that fetches data from a reachable API. We created a Model to provide the data, a ViewModel to manage the data, and a View to display the data. By using MVVM, we separated the user interface logic from the business logic, making our code more scalable and testable. MVVM is a powerful design pattern that can be used in a variety of applications. By mastering it, you can build robust and maintainable apps that are easy to maintain and modify.