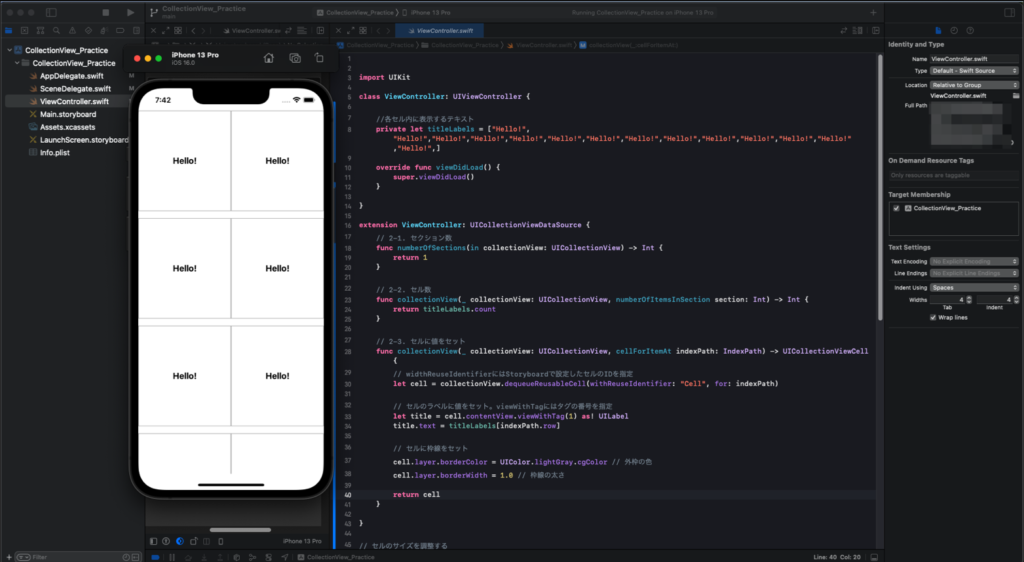
Storyboard
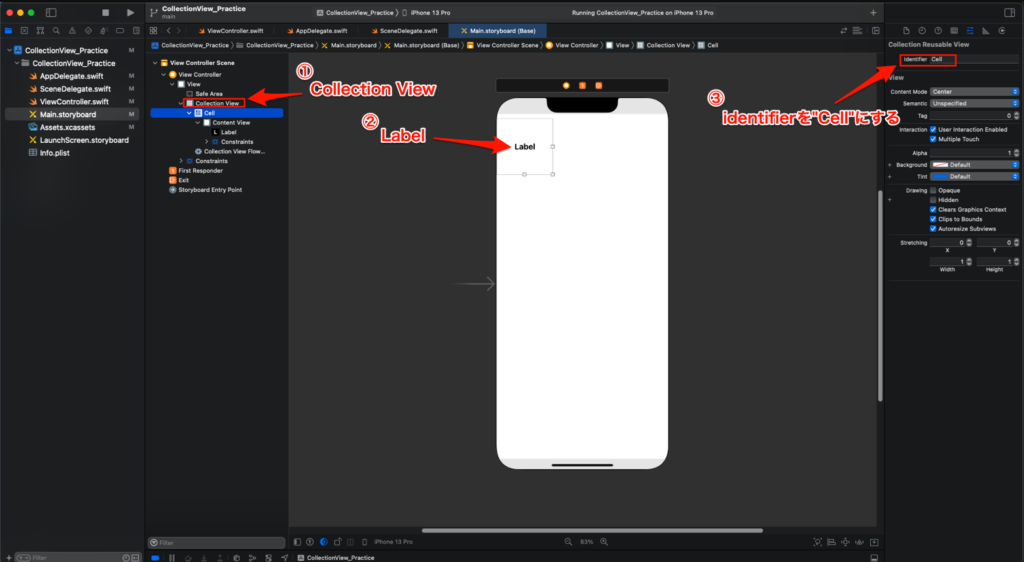
今回はLabelにタグをつけておいてください。
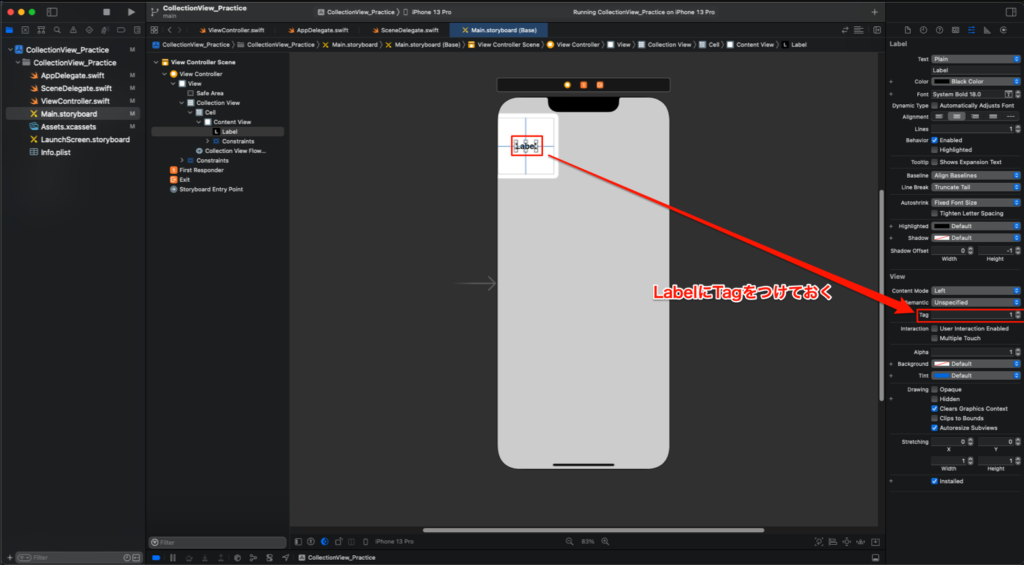
最後にDelegateとDatasourceをViewControllerに繋げる
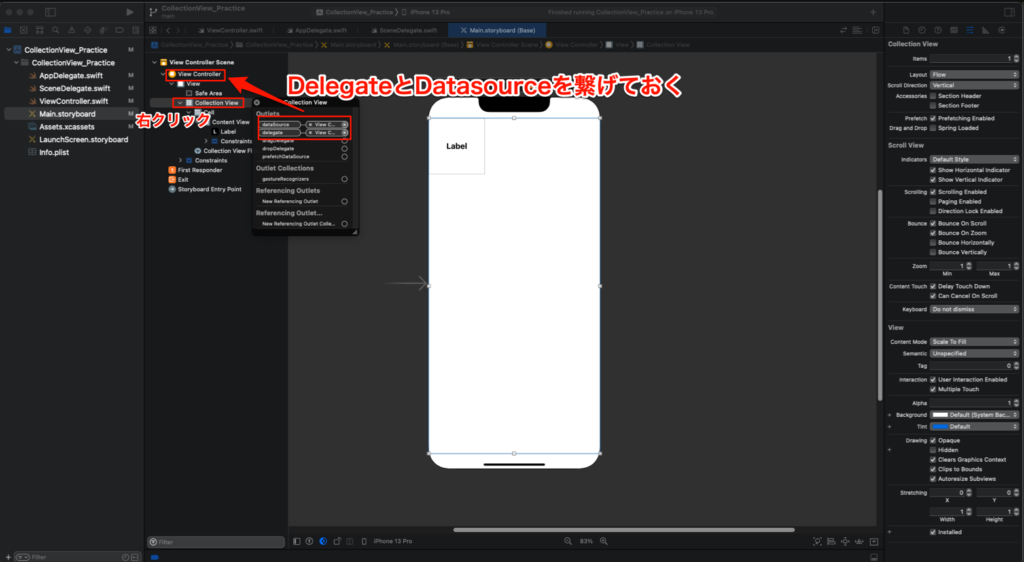
コード記述
import UIKit
class ViewController: UIViewController {
//各セル内に表示するテキスト
private let titleLabels = ["Hello!", "Hello!","Hello!","Hello!","Hello!","Hello!","Hello!","Hello!","Hello!","Hello!","Hello!","Hello!","Hello!","Hello!"]
override func viewDidLoad() {
super.viewDidLoad()
}
}
extension ViewController: UICollectionViewDataSource {
// 2-1. セクション数
func numberOfSections(in collectionView: UICollectionView) -> Int {
return 1
}
// 2-2. セル数
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return titleLabels.count
}
// 2-3. セルに値をセット
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
// widthReuseIdentifierにはStoryboardで設定したセルのIDを指定
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "Cell", for: indexPath)
// セルのラベルに値をセット。viewWithTagにはタグの番号を指定
let title = cell.contentView.viewWithTag(1) as! UILabel
title.text = titleLabels[indexPath.row]
// セルに枠線をセット
cell.layer.borderColor = UIColor.lightGray.cgColor // 外枠の色
cell.layer.borderWidth = 1.0 // 枠線の太さ
return cell
}
}
// セルのサイズを調整する
extension ViewController: UICollectionViewDelegateFlowLayout {
// セルサイズを指定する
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
// 横方向のサイズを調整
let cellSizeWidth:CGFloat = self.view.frame.width/2
let cellSizeHeight:CGFloat = self.view.frame.height/2
// widthとheightのサイズを返す
return CGSize(width: cellSizeWidth, height: cellSizeHeight/2)
}
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, minimumLineSpacingForSectionAt section: Int) -> CGFloat {
return 15.0 // 行間
}
}
各セルの背景色や間隔の開け方等詳細設定も出来るのでぜひ調べながらカスタマイズしてみてください (丸投げ)
この記事は役に立ちましたか?
貴重なフィードバックありがとうございます!