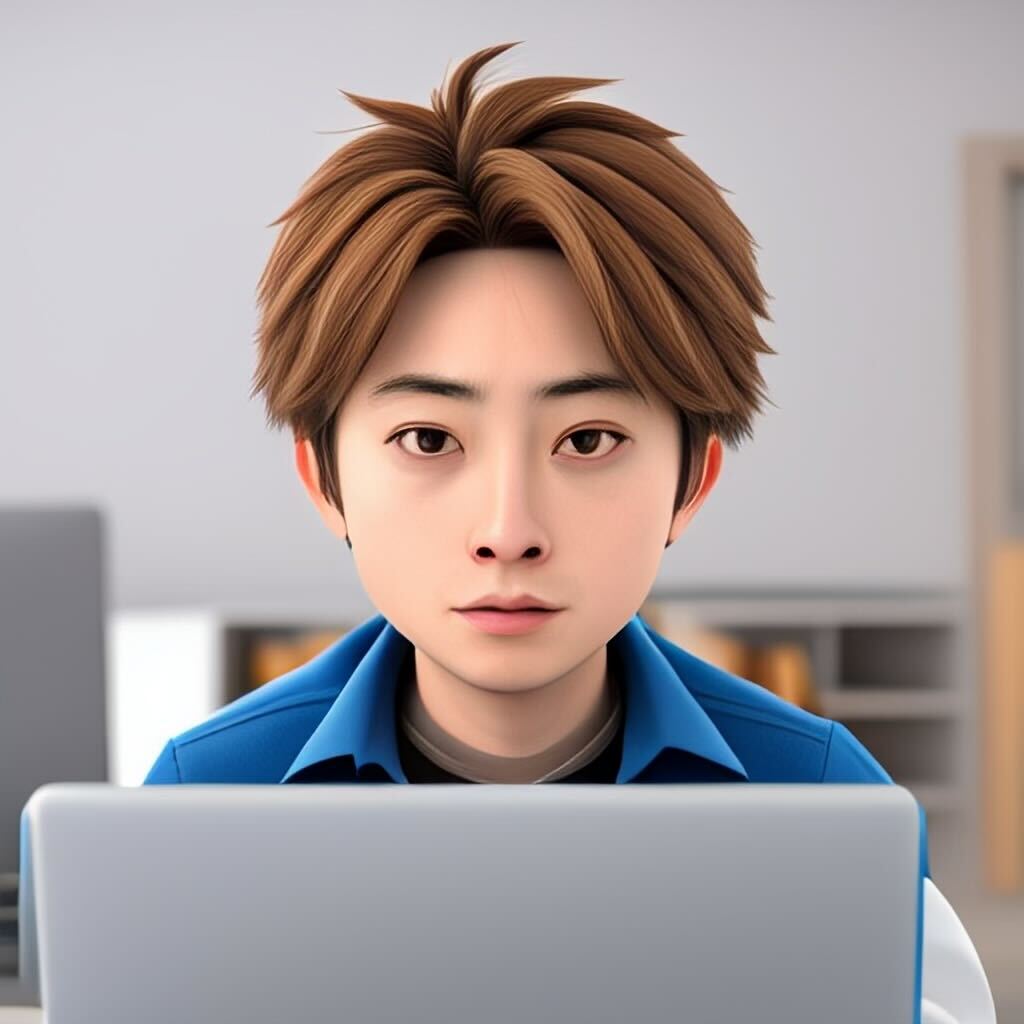
今回はCellをロングタップした時に小さいウィンドウの選択画面を開く実装方法を共有します。
簡単に取り入れられるので便利です。
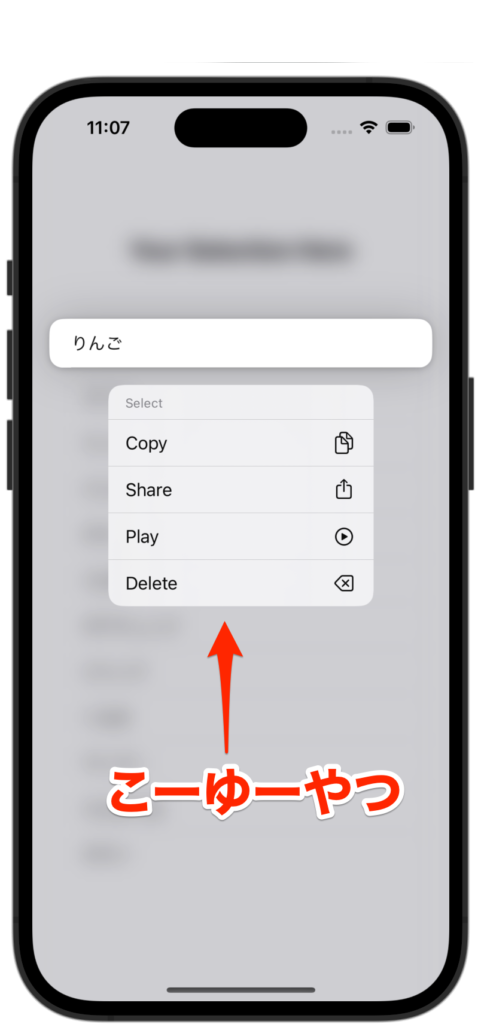
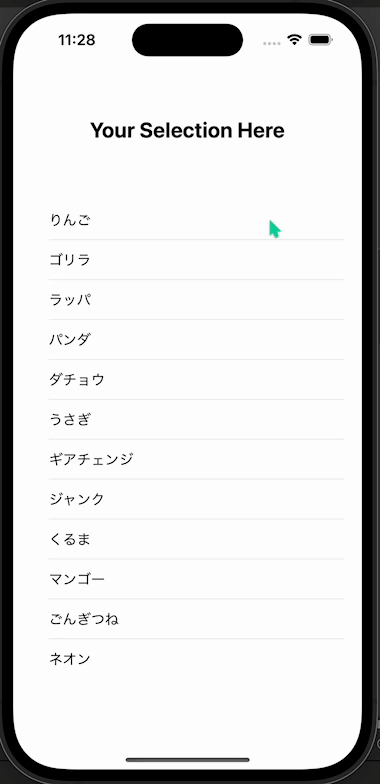
Contents 非表示
Storyboard
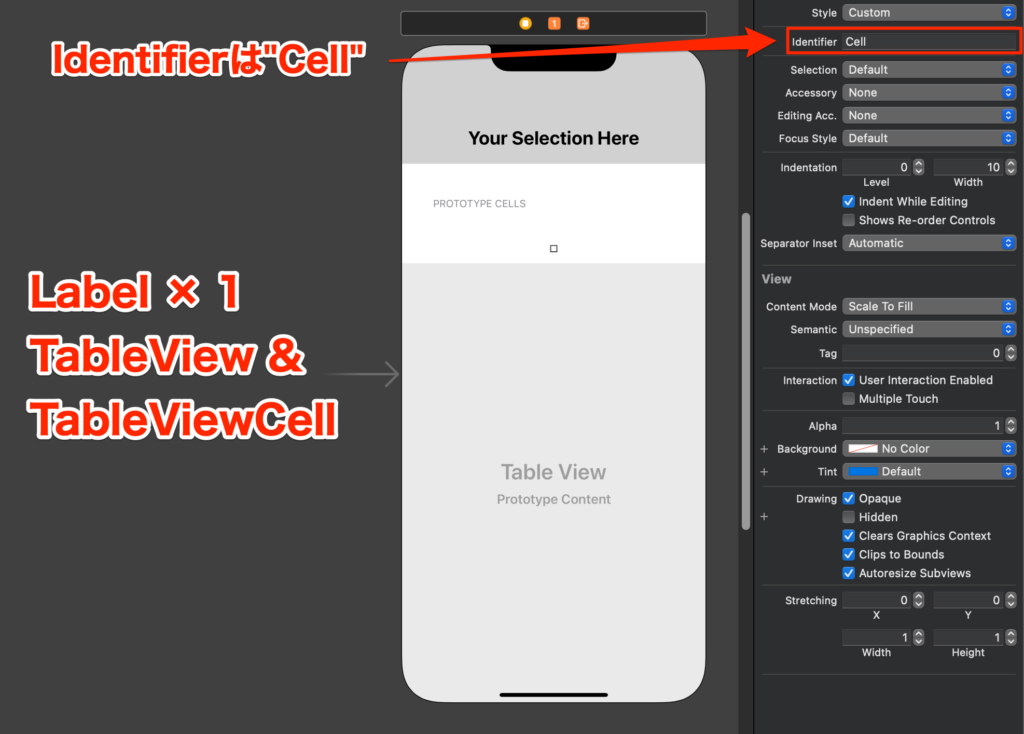
コーディング
import UIKit
class HomeViewController: UIViewController {
@IBOutlet private weak var titleLabel: UILabel!
@IBOutlet private weak var tableView: UITableView!
private let sampleArray: [String] = ["りんご", "ゴリラ", "ラッパ", "パンダ", "ダチョウ", "うさぎ", "ギアチェンジ", "ジャンク", "くるま", "マンゴー", "ごんぎつね", "ネオン"]
override func viewDidLoad() {
super.viewDidLoad()
setupTableView()
}
private func setupTableView() {
tableView.delegate = self
tableView.dataSource = self
}
// ContextMenuに表示するもの
private func createContextMenu() -> UIMenu {
let copy = UIAction(title: "Copy", image: UIImage(systemName: "doc.on.doc")) { action in
self.titleLabel.text = "Copyだよ"
}
let share = UIAction(title: "Share", image: UIImage(systemName: "square.and.arrow.up")) { action in
self.titleLabel.text = "Shareだよ"
}
let play = UIAction(title: "Play", image: UIImage(systemName: "play.circle")) { action in
self.titleLabel.text = "Playだよ"
}
let delete = UIAction(title: "Delete", image: UIImage(systemName: "delete.left")) { action in
self.titleLabel.text = "Deleteだよ"
}
return UIMenu(title: "Select", children: [copy, share, play, delete])
}
}
extension HomeViewController: UITableViewDelegate {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return sampleArray.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath)
cell.textLabel?.text = sampleArray[indexPath.row]
return cell
}
}
extension HomeViewController: UITableViewDataSource {
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
tableView.deselectRow(at: indexPath, animated: true)
}
func tableView(_ tableView: UITableView, contextMenuConfigurationForRowAt indexPath: IndexPath, point: CGPoint) -> UIContextMenuConfiguration? {
return UIContextMenuConfiguration(identifier: nil, previewProvider: nil, actionProvider: { suggestedActions in
return self.createContextMenu()
})
}
}
まとめ
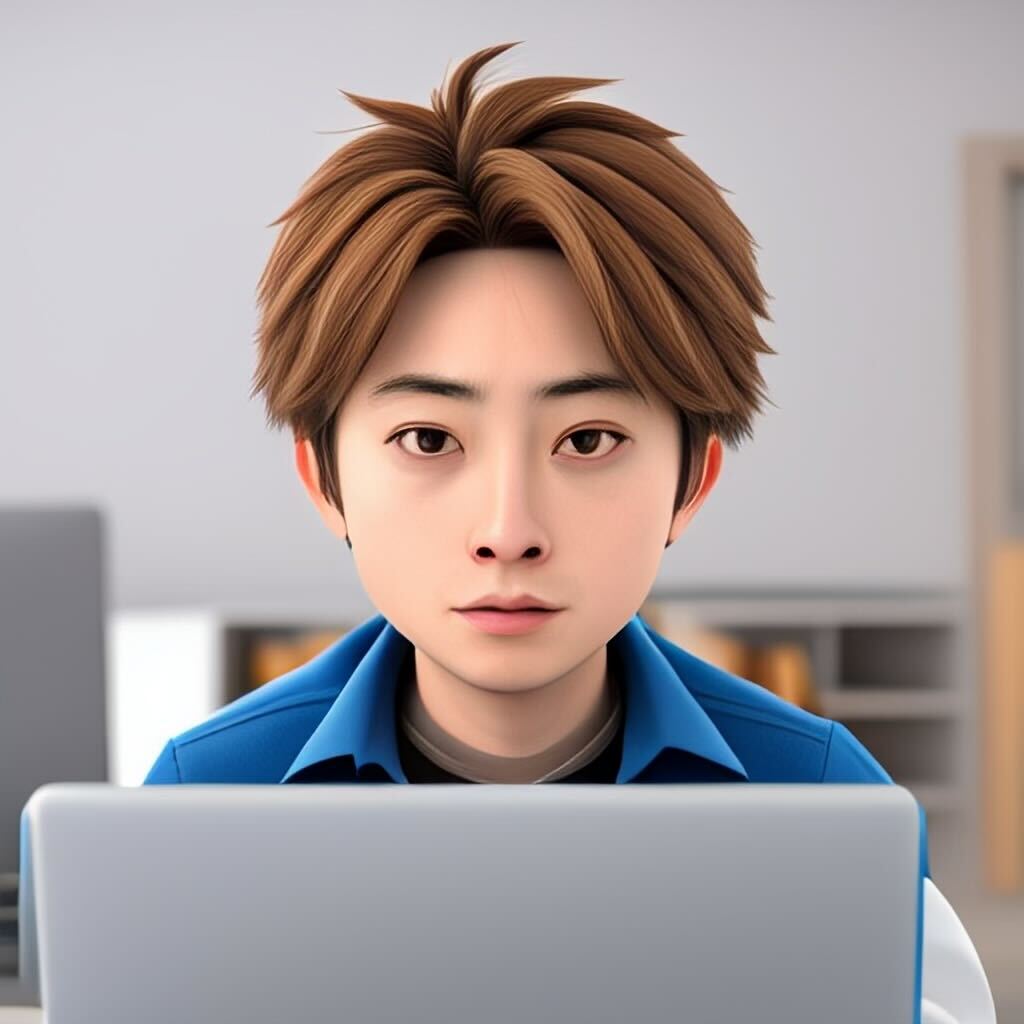
今回は以上です、シンプルな実装でも使い方は無限大なので自分なりにカスタマイズしていきましょう!
◎今日の格言
ー John.F. Kennedy
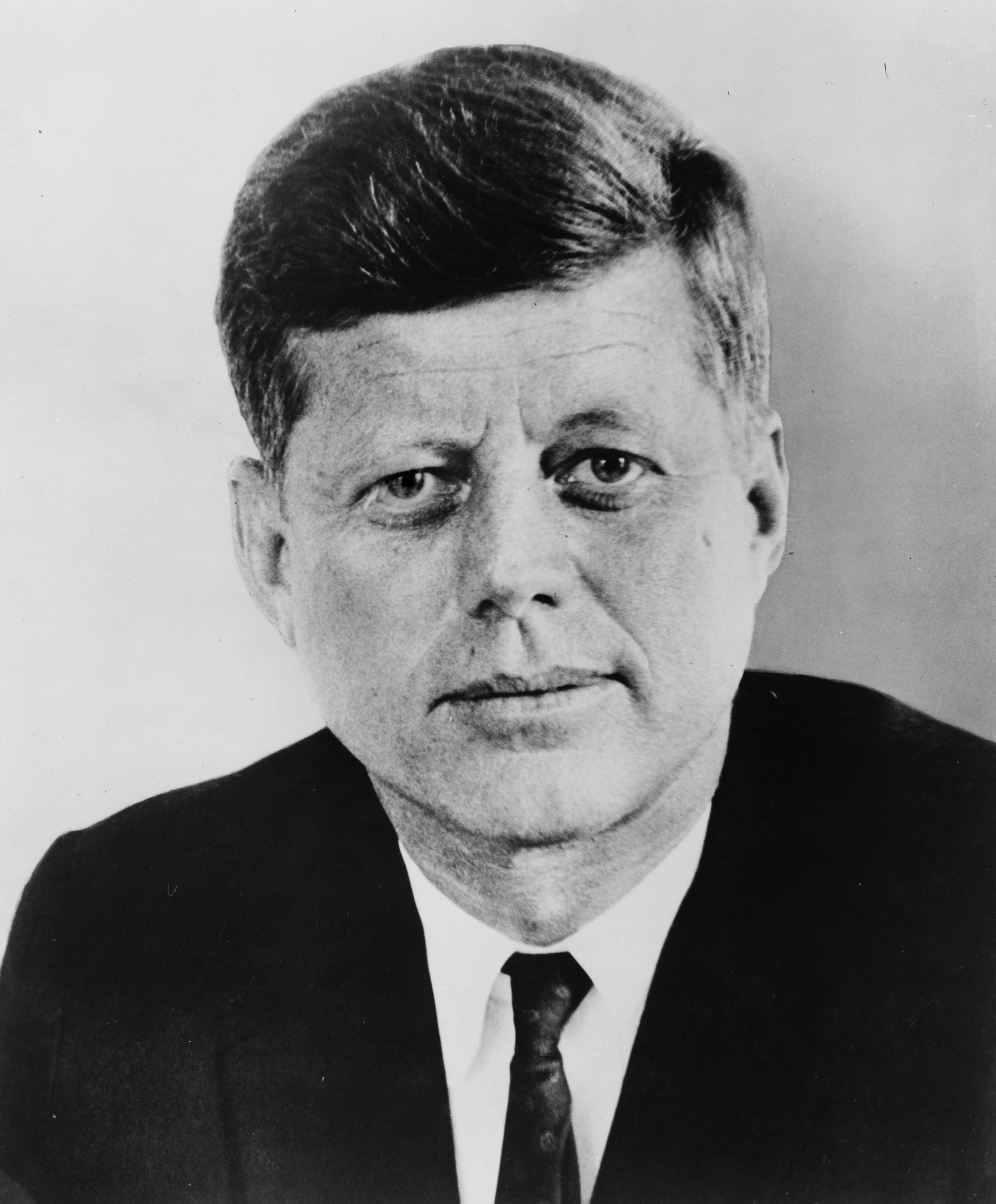
John
Leadership and learning are indispensable to each other.
(“リーダーシップ”と”学ぶ姿勢”は互いに持ち合わせていなければならないものだ)