今回の内容
・画面スワイプでモーダル遷移をさせる方法を学ぶ
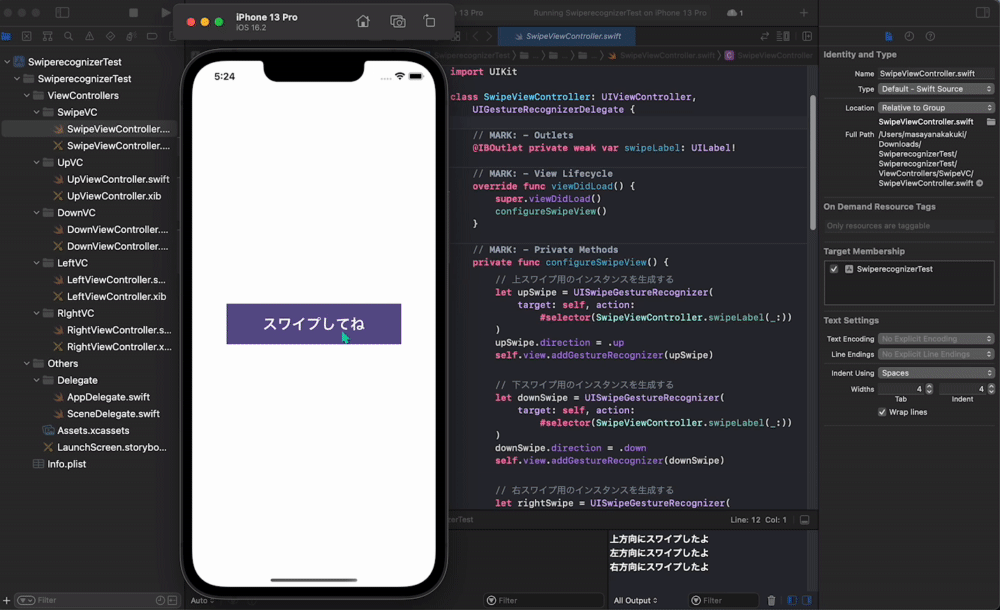
プロジェクト構成
* 画像ではXib開発ですが普通にStoryboardのまま進めてOKです
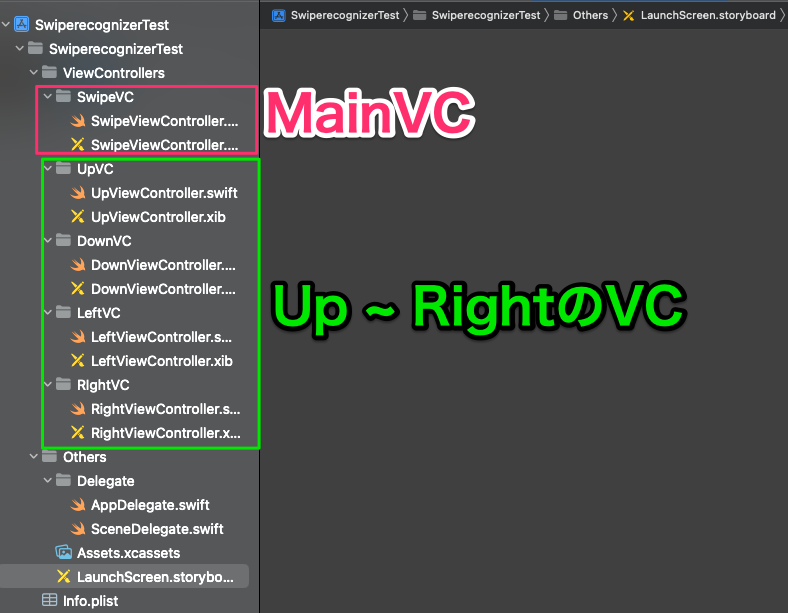
Storyboard
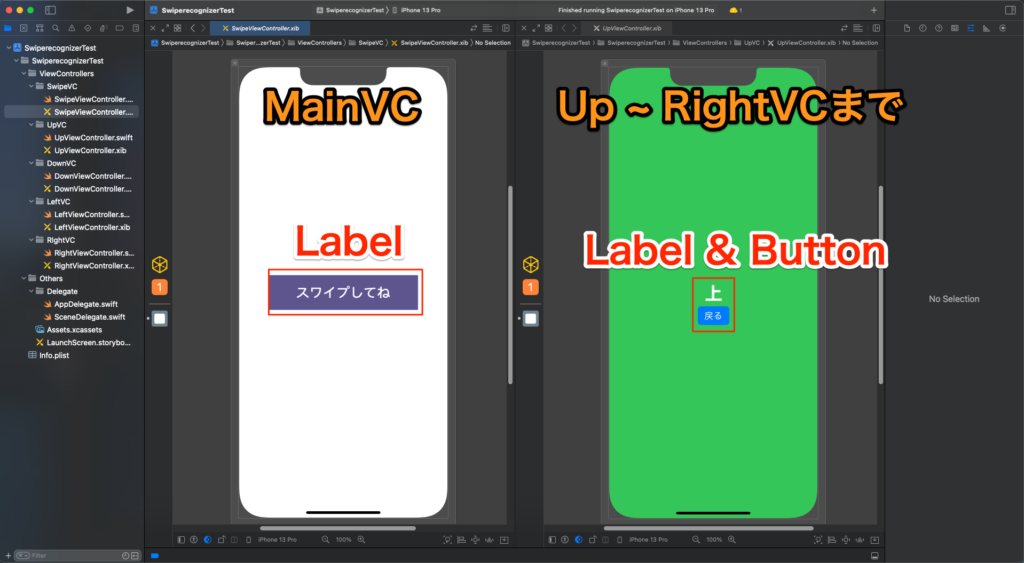
コード
MainViewController
import UIKit
class SwipeViewController: UIViewController, UIGestureRecognizerDelegate {
// MARK: - Outlets
@IBOutlet private weak var swipeLabel: UILabel!
// MARK: - View Lifecycle
override func viewDidLoad() {
super.viewDidLoad()
configureSwipeView()
}
// MARK: - Private Methods
private func configureSwipeView() {
// 上スワイプ用のインスタンスを生成する
let upSwipe = UISwipeGestureRecognizer(
target: self, action: #selector(SwipeViewController.swipeLabel(_:))
)
upSwipe.direction = .up
self.view.addGestureRecognizer(upSwipe)
// 下スワイプ用のインスタンスを生成する
let downSwipe = UISwipeGestureRecognizer(
target: self, action: #selector(SwipeViewController.swipeLabel(_:))
)
downSwipe.direction = .down
self.view.addGestureRecognizer(downSwipe)
// 右スワイプ用のインスタンスを生成する
let rightSwipe = UISwipeGestureRecognizer(
target: self, action: #selector(SwipeViewController.swipeLabel(_:))
)
rightSwipe.direction = .right
self.view.addGestureRecognizer(rightSwipe)
// 左スワイプ用のインスタンスを生成する
let leftSwipe = UISwipeGestureRecognizer(
target: self, action: #selector(SwipeViewController.swipeLabel(_:))
)
leftSwipe.direction = .left
self.view.addGestureRecognizer(leftSwipe)
}
// スワイプした方向をラベルに表示する。
@objc private func swipeLabel(_ sender: UISwipeGestureRecognizer) {
switch sender.direction {
case UISwipeGestureRecognizer.Direction.up:
let upViewController = UpViewController(nibName: "UpViewController", bundle: nil)
// upViewController.modalPresentationStyle = .fullScreen // フルスクリーンモーダル遷移の場合
print("上方向にスワイプしたよ")
self.present(upViewController, animated: true, completion: nil)
case UISwipeGestureRecognizer.Direction.down:
let downViewController = DownViewController(nibName: "DownViewController", bundle: nil)
// downViewController.modalPresentationStyle = .fullScreen // フルスクリーンモーダル遷移の場合
print("下方向にスワイプしたよ")
self.present(downViewController, animated: true, completion: nil)
case UISwipeGestureRecognizer.Direction.left:
let leftViewController = LeftViewController(nibName: "LeftViewController", bundle: nil)
// leftViewController.modalPresentationStyle = .fullScreen // フルスクリーンモーダル遷移の場合
print("左方向にスワイプしたよ")
self.present(leftViewController, animated: true, completion: nil)
case UISwipeGestureRecognizer.Direction.right:
let rightViewController = RightViewController(nibName: "RightViewController", bundle: nil)
// rightViewController.modalPresentationStyle = .fullScreen // フルスクリーンモーダル遷移の場合
print("右方向にスワイプしたよ")
self.present(rightViewController, animated: true, completion: nil)
default:
break
}
}
}
Up ~ RightのViewController
import UIKit
class <#方向#>ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
}
@IBAction func backAction(_ sender: Any) {
dismiss(animated: true)
}
}