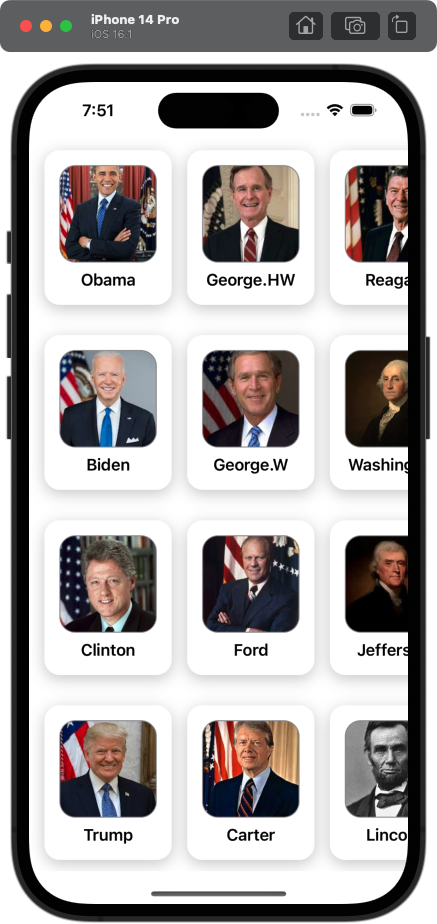
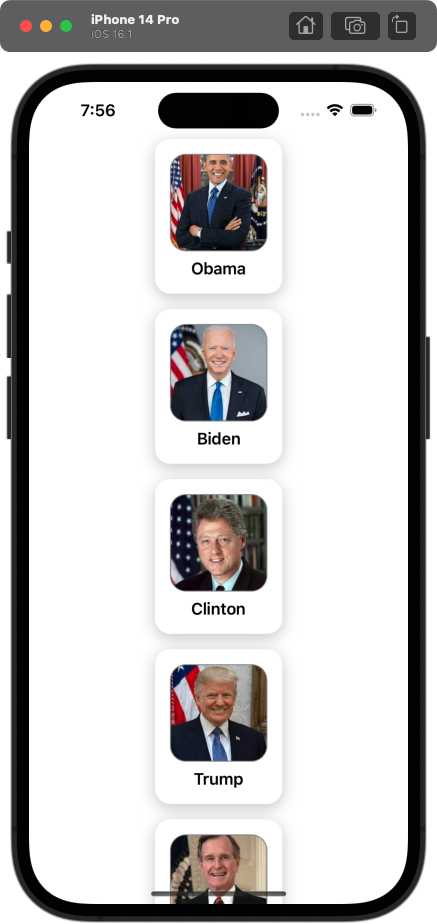
Contents 非表示
実装
Item
import Foundation
import SwiftUI
struct Item: Identifiable {
let id = UUID()
let imageName: String
let title: String
}
struct FancyGridItem: View {
let item: Item
var body: some View {
VStack(spacing: 8) {
Image(item.imageName)
.resizable()
.aspectRatio(contentMode: .fill)
.frame(width: 100, height: 100)
.cornerRadius(16)
.overlay(
RoundedRectangle(cornerRadius: 16)
.stroke(Color.gray, lineWidth: 1)
)
Text(item.title)
.font(.headline)
.foregroundColor(.primary)
}
.padding(16)
.background(Color.white)
.cornerRadius(16)
.shadow(color: Color.black.opacity(0.2), radius: 8, x: 0, y: 4)
}
}
HomeView (メインのView)
import SwiftUI
struct HomeView: View {
private let items: [Item] = [
Item(imageName: "img_barack", title: "Obama"),
Item(imageName: "img_biden", title: "Biden"),
Item(imageName: "img_bill", title: "Clinton"),
Item(imageName: "img_donald", title: "Trump"),
Item(imageName: "img_george_hw", title: "George.HW"),
Item(imageName: "img_george_w", title: "George.W"),
Item(imageName: "img_gerald", title: "Ford"),
Item(imageName: "img_jimmy", title: "Carter"),
Item(imageName: "img_ronald", title: "Reagan"),
Item(imageName: "img_washington", title: "Washington"),
Item(imageName: "img_thomas", title: "Jefferson"),
Item(imageName: "img_abraham", title: "Lincoln")
]
private let gridItems = [GridItem(.adaptive(minimum: 180))]
var body: some View {
ScrollView(.horizontal) {
LazyHGrid(rows: gridItems, spacing: 16) {
ForEach(items) { item in
FancyGridItem(item: item)
}
}
.padding(.horizontal, 16)
}
// LazyVGrid
// ScrollView(.vertical) {
// LazyVGrid(columns: gridItems, spacing: 16) {
// ForEach(items) { item in
// FancyGridItem(item: item)
// }
// }
// .padding(.horizontal, 16)
// }
}
}