Contents 非表示
SwiftGenとは?
プロジェクト内で使用する色、画像、フォントのようなリソース名を自動的に生成して、型付けが出来るライブラリです。
これによってタイポや存在しないAssetsを使用する等のリスクをなくすことが出来ます。
インストール
pod 'SwiftGen', '~> 6.0'
設定ファイル
touch swiftgen.yml
で、ファイルを追加する。
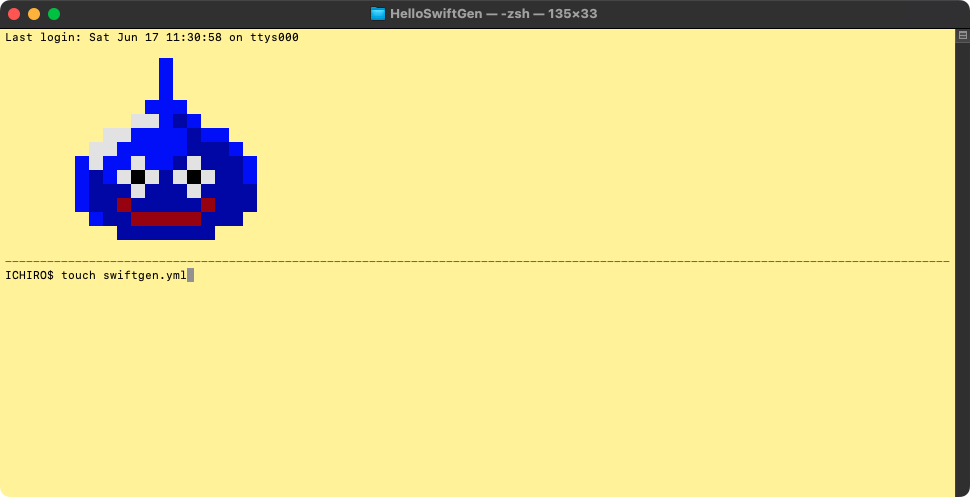
作れたらファイルを開いて以下の様に記述
xcassets:
inputs:
- HelloSwiftGen/Resources/Assets.xcassets
- HelloSwiftGen/Resources/Colors.xcassets
outputs:
templateName: swift5
output: HelloSwiftGen/Generated/Assets.swift
strings:
inputs: HelloSwiftGen/Resources/Localizable.strings
outputs:
templateName: structured-swift5
output: HelloSwiftGen/Generated/LocalizableStrings.swift
fonts:
inputs: HelloSwiftGen/Resources/Font
outputs:
templateName: swift5
output: HelloSwiftGen/Generated/Fonts.swift
HelloSwiftGen
の部分は自身のプロジェクト名に置換してください。
ファイルの追加
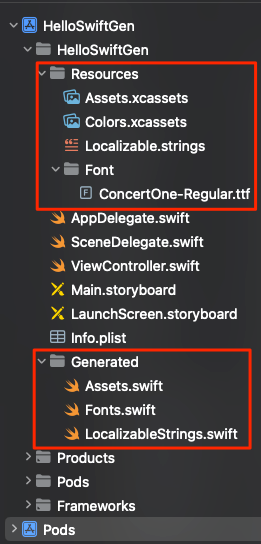
赤枠で囲った箇所が重要です。
- プロジェクトフォルダ直下に
Resources
、Generated
というフォルダを作成 Resources
フォルダ内には、①Assets.xcassets
、②Colors.xcassets
、③Localizable.strings
、④Font
フォルダを入れる (②③④は新規作成する必要あり)Font
フォルダ内にフォントファイルを入れる、GoogleFontとかから持ってくればOKGenerated
フォルダ内には、①Assets.swift
、②Fonts.swift
、③LocalizableStrings.swift
を入れる
各リソースを入れる
Assets.xcassets
には画像を、Colors.xcassets
にはカラーを、Localizable.strings
にはテキストを入れる。
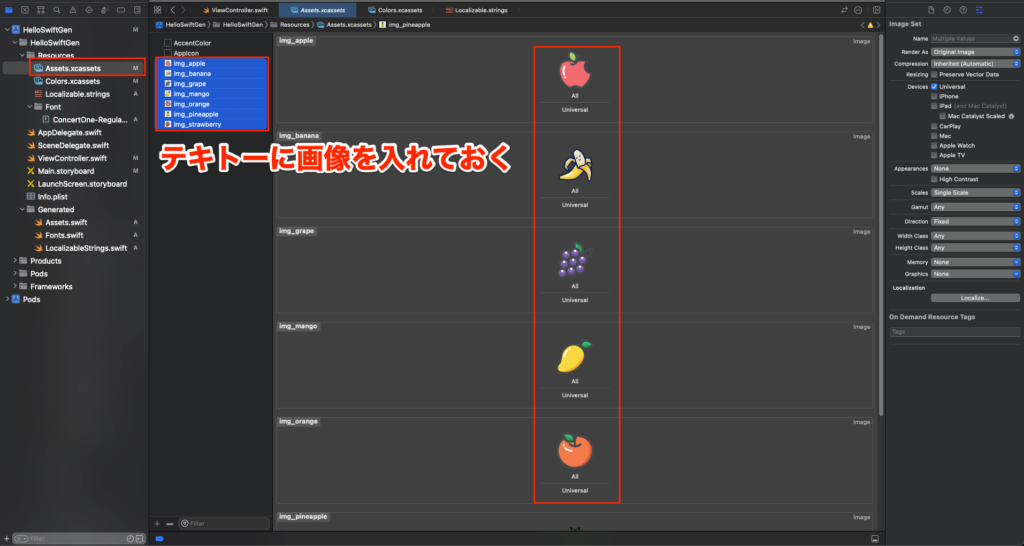
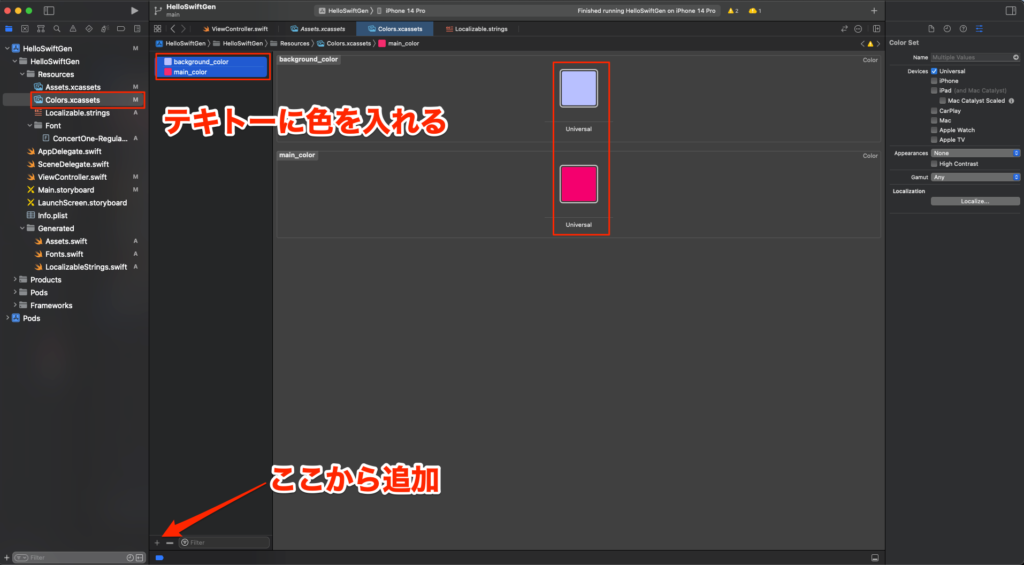
// sample
"sample_text1" = "A rose by any other name would smell as sweet.";
"sample_text2" = "Ask not what your country can do for you; ask what you can do for your country.";
"sample_text3" = "Ask, and it shall be given you; seek, and you shall find.";
"sample_text4" = "Genius is one percent inspiration and ninety-nine percent perspiration.";
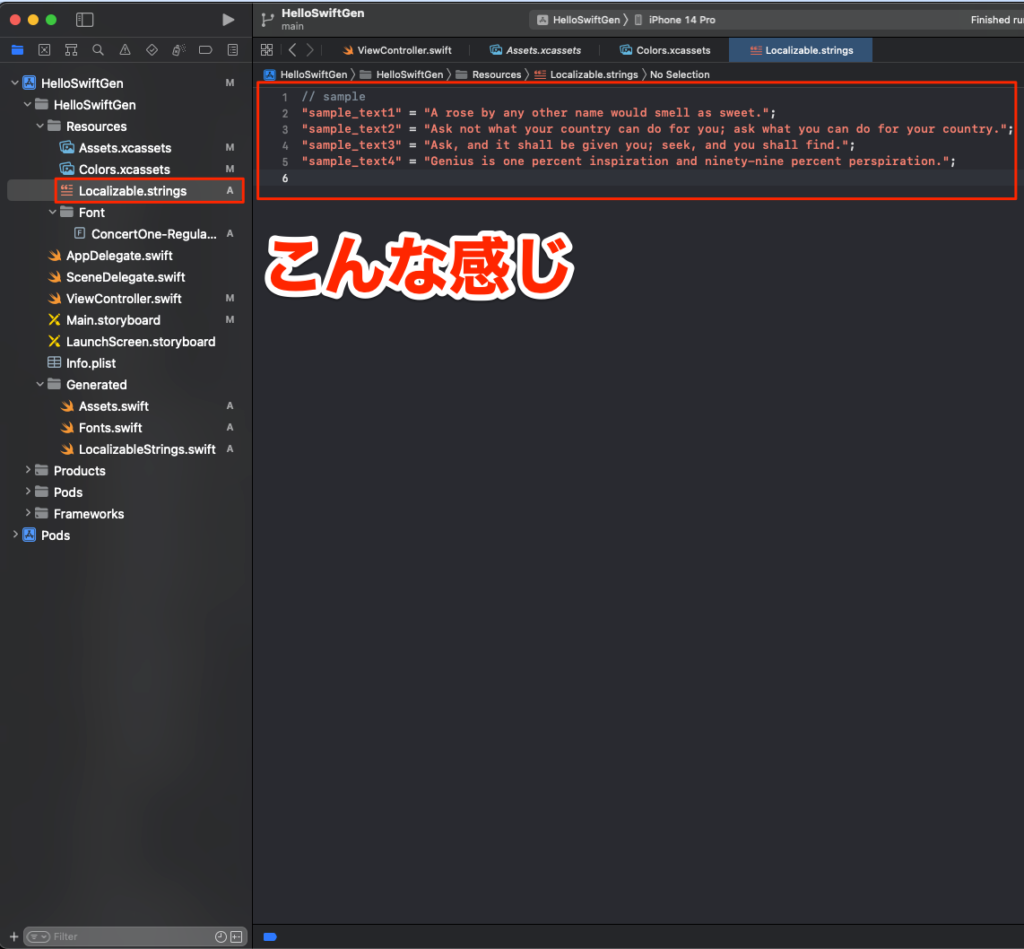
最終チェック、コマンド実行
swiftgen config lint
でステータスを確認する、エラーが出てなければOK
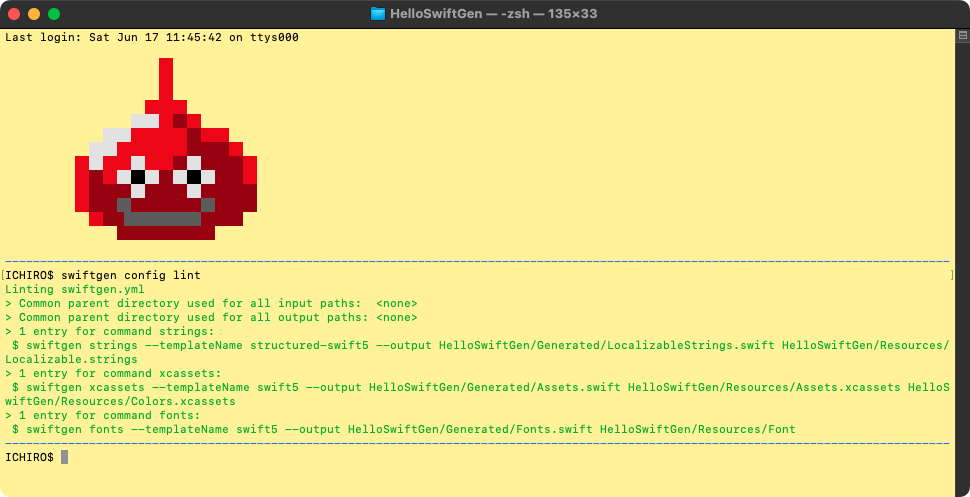
問題なければswiftgen config run
を実行、これで自動補完が可能になります。
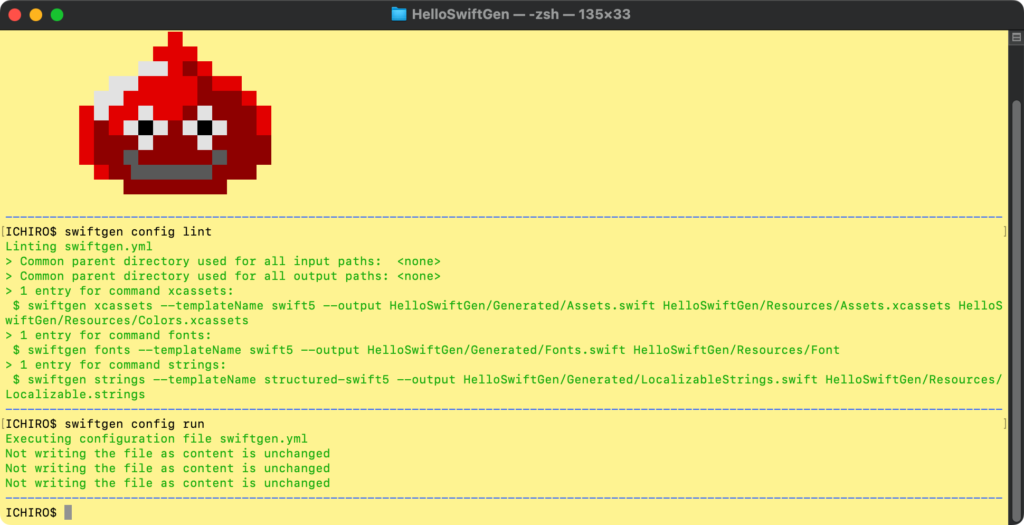
サンプル実装
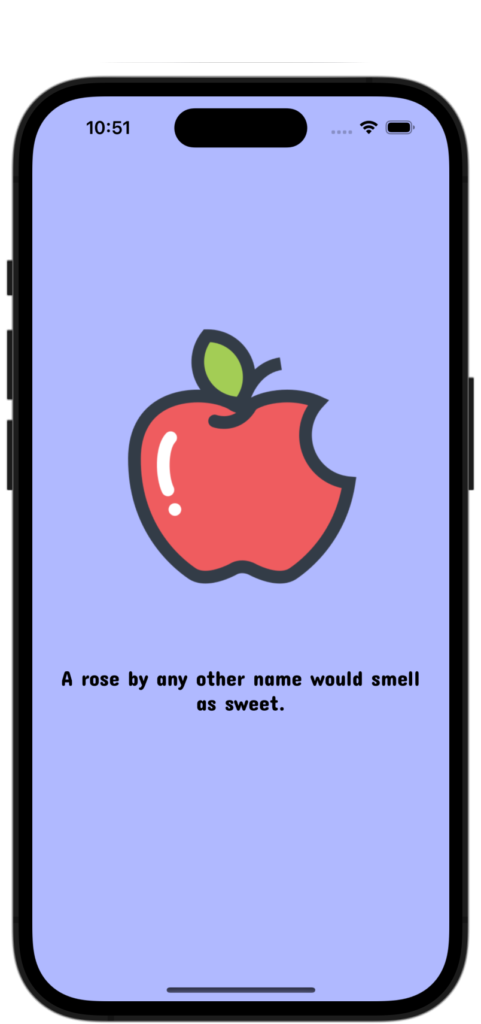
Storyboard
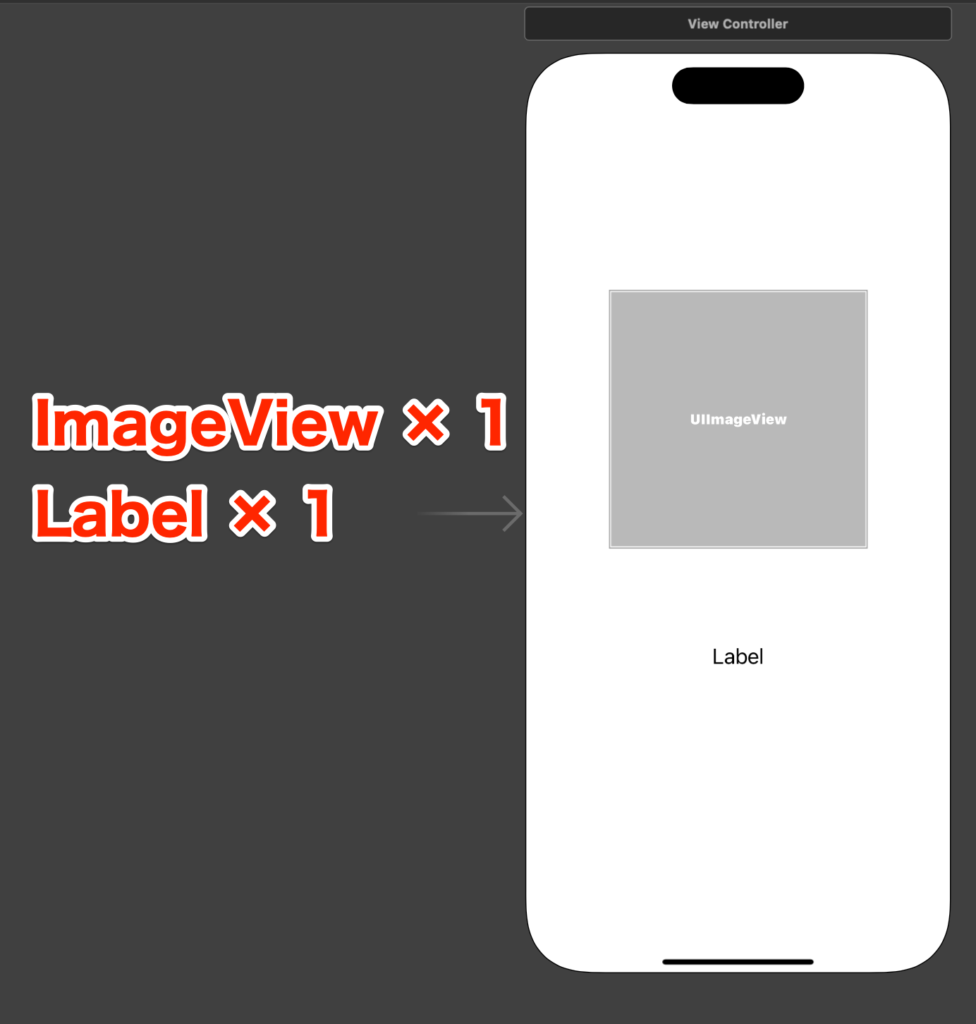
コーディング
import UIKit
class ViewController: UIViewController {
@IBOutlet private weak var imageView: UIImageView!
@IBOutlet private weak var label: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
// SwiftGenを使う場合
self.view.backgroundColor = Asset.Colors.backgroundColor.color
imageView.image = Asset.Assets.imgApple.image
label.text = L10n.sampleText1
label.font = FontFamily.ConcertOne.regular.font(size: 20)
// SwiftGenを使わない場合
// self.view.backgroundColor = .blue
// imageView.image = UIImage(named: "img_apple")
// label.text = "A rose by any other name would smell as sweet."
// label.font = UIFont(font: "ConcertOne-Regular", size: 20)
}
}
そのままだとimageView
のnameやtext
でのタイポがある可能性がありますが、SwiftGen
を使用することでリソースの型付けが出来るため、うっかりミスを防ぐことが出来ます。