
MARKコメントを使うことでコードの可読性を上げることができます、ぜひ使ってみましょう
サンプルプロジェクト
テキトーにTableViewに何かを表示させてみる
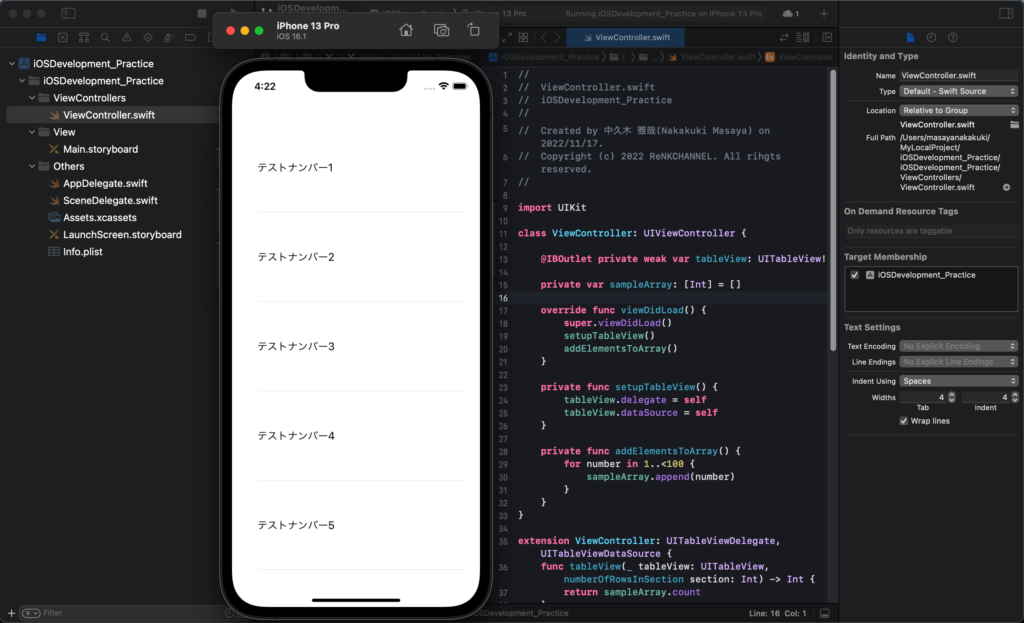
import UIKit
class ViewController: UIViewController {
@IBOutlet private weak var tableView: UITableView!
private var sampleArray: [Int] = []
override func viewDidLoad() {
super.viewDidLoad()
setupTableView()
addElementsToArray()
}
private func setupTableView() {
tableView.delegate = self
tableView.dataSource = self
}
private func addElementsToArray() {
for number in 1..<100 {
sampleArray.append(number)
}
}
}
extension ViewController: UITableViewDelegate, UITableViewDataSource {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return sampleArray.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath)
cell.textLabel?.text = "テストナンバー\(sampleArray[indexPath.row])"
return cell
}
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return self.view.frame.height / 6
}
}
今回のサンプルコードは元がシンプルなので特にコードが読みづらいということは起こりづらいですが、これがもっと複雑になってくるとどこで何が定義されているのかなどが分かりづらくなってきます。
そんなときにMARKコメントで区切りをつけることで区切り線が作られて可読性をグッと高めることが可能になります。
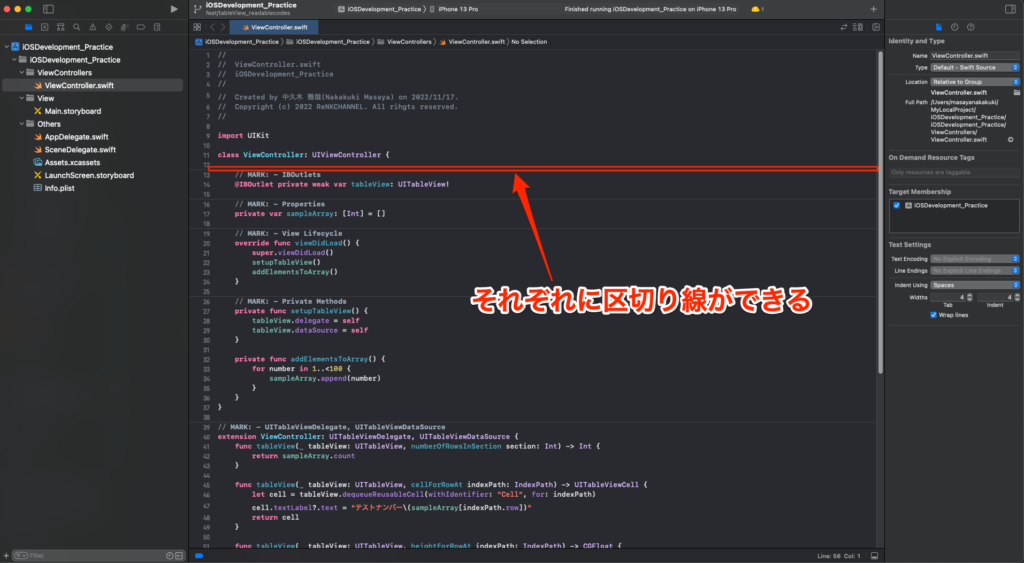
import UIKit
class ViewController: UIViewController {
// MARK: - IBOutlets
@IBOutlet private weak var tableView: UITableView!
// MARK: - Properties
private var sampleArray: [Int] = []
// MARK: - View Lifecycle
override func viewDidLoad() {
super.viewDidLoad()
setupTableView()
addElementsToArray()
}
// MARK: - Private Methods
private func setupTableView() {
tableView.delegate = self
tableView.dataSource = self
}
private func addElementsToArray() {
for number in 1..<100 {
sampleArray.append(number)
}
}
}
// MARK: - UITableViewDelegate, UITableViewDataSource
extension ViewController: UITableViewDelegate, UITableViewDataSource {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return sampleArray.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath)
cell.textLabel?.text = "テストナンバー\(sampleArray[indexPath.row])"
return cell
}
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return self.view.frame.height / 6
}
}
まとめ (るほどでもない)

チーム開発をしていく際にはもちろん自分以外にもコードを見る人がいるので誰が見ても分かりやすいコード書くことを心がけていきましょう。