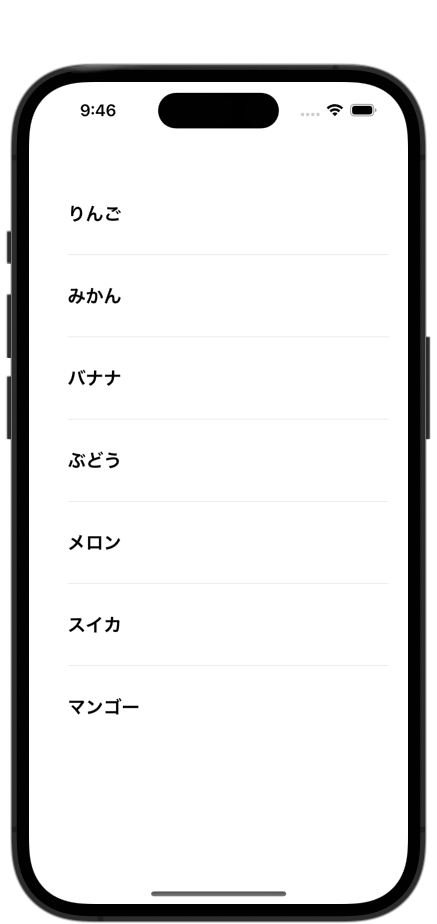
Contents 非表示
実装
View側
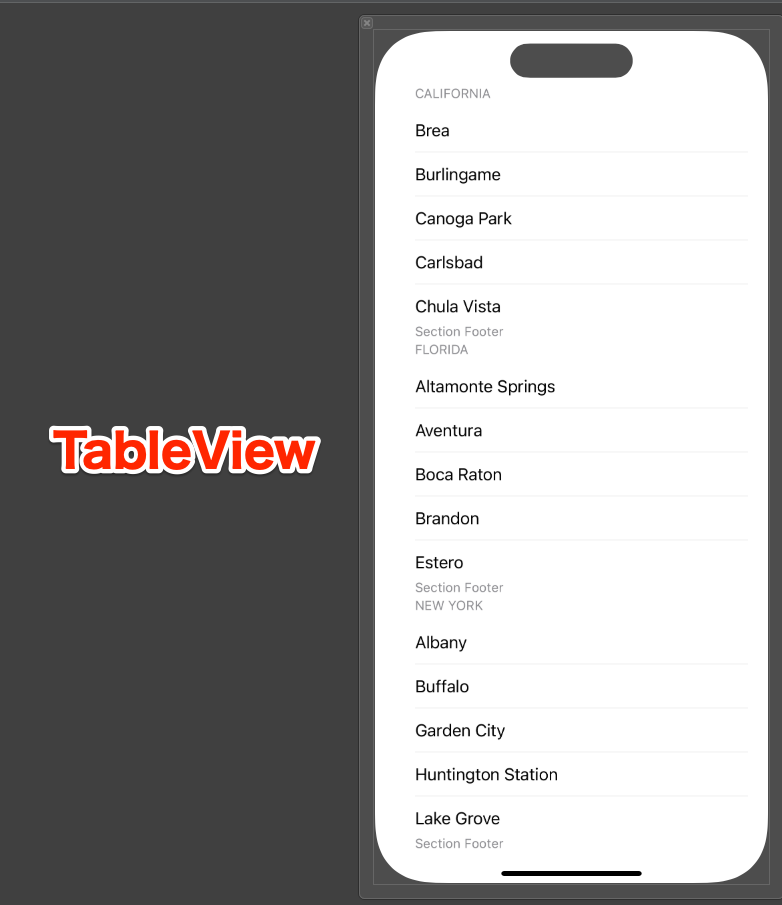
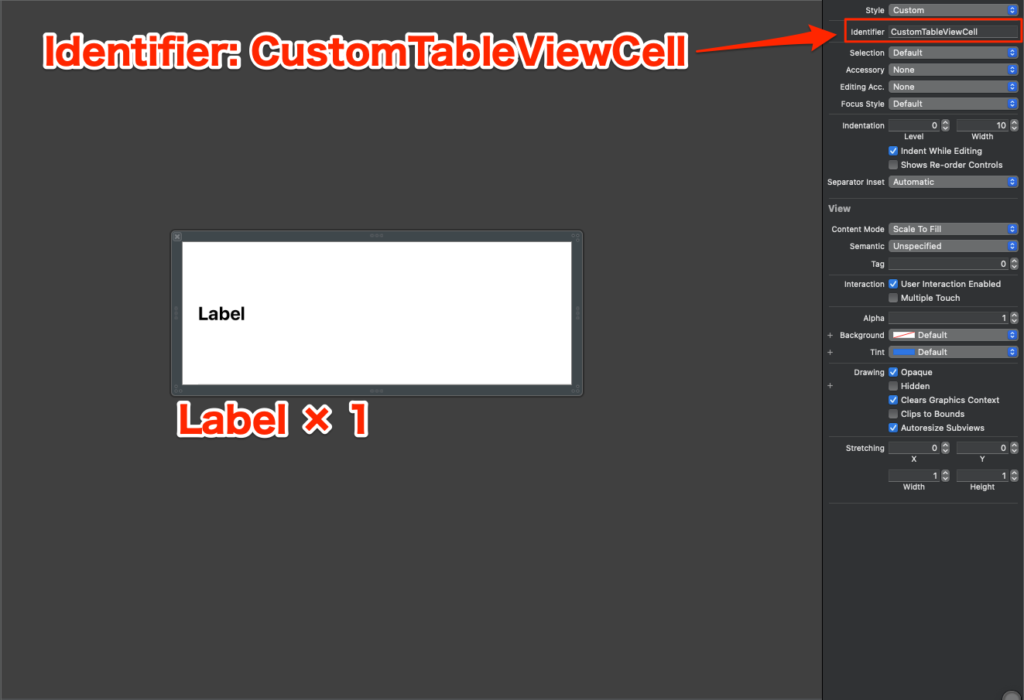
ロジック側
import UIKit
final class ViewController: UIViewController {
// MARK: - Properties
@IBOutlet private weak var tableView: UITableView!
private let fruitsArray: [String] = ["りんご", "みかん", "バナナ", "ぶどう", "メロン", "スイカ", "マンゴー"]
// MARK: - View Life Cycle
override func viewDidLoad() {
super.viewDidLoad()
setupTableView()
}
private func setupTableView() {
tableView.delegate = self
tableView.dataSource = self
tableView.register(UINib(nibName: "CustomTableViewCell", bundle: nil), forCellReuseIdentifier: "CustomTableViewCell")
}
}
// MARK: - Extensions
extension ViewController: UITableViewDelegate, UITableViewDataSource {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return fruitsArray.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
guard let cell = tableView.dequeueReusableCell(withIdentifier: "CustomTableViewCell", for: indexPath) as? CustomTableViewCell else {
return UITableViewCell()
}
cell.configure(fruitsArray[indexPath.row])
return cell
}
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return self.view.frame.height / 10
}
}
import UIKit
class CustomTableViewCell: UITableViewCell {
@IBOutlet private weak var label: UILabel!
override func prepareForReuse() {
super.prepareForReuse()
label.text = nil
}
func configure(_ name: String) {
label.text = name
}
}