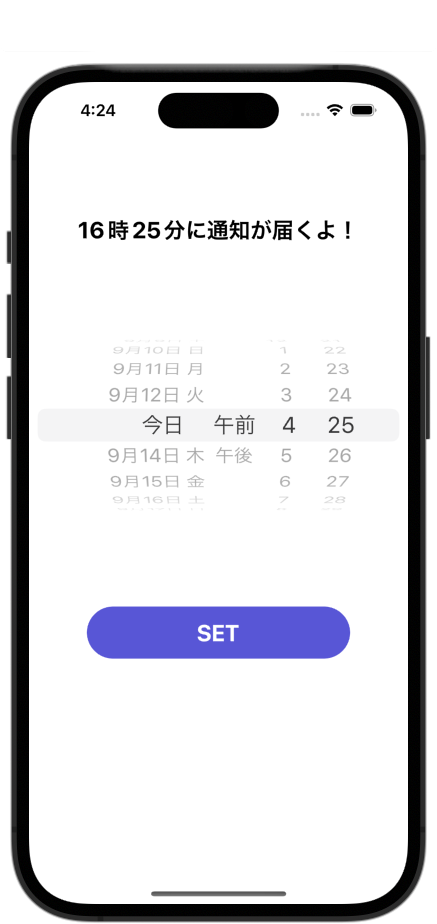
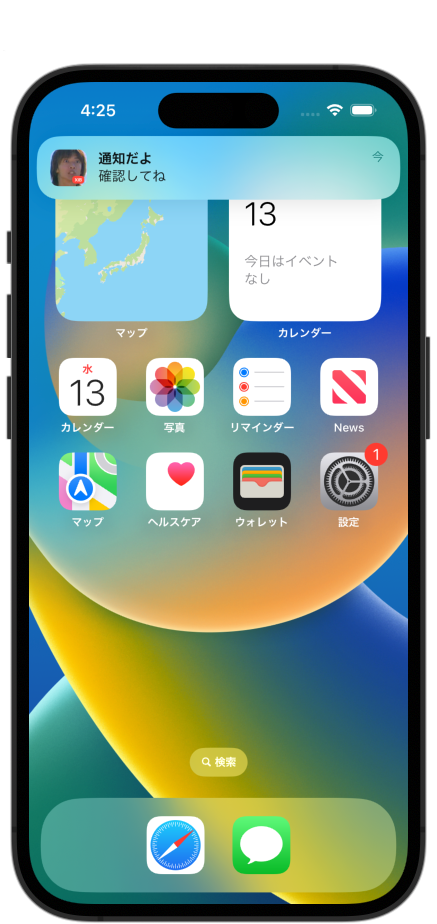
Contents 非表示
実装
Storyboard
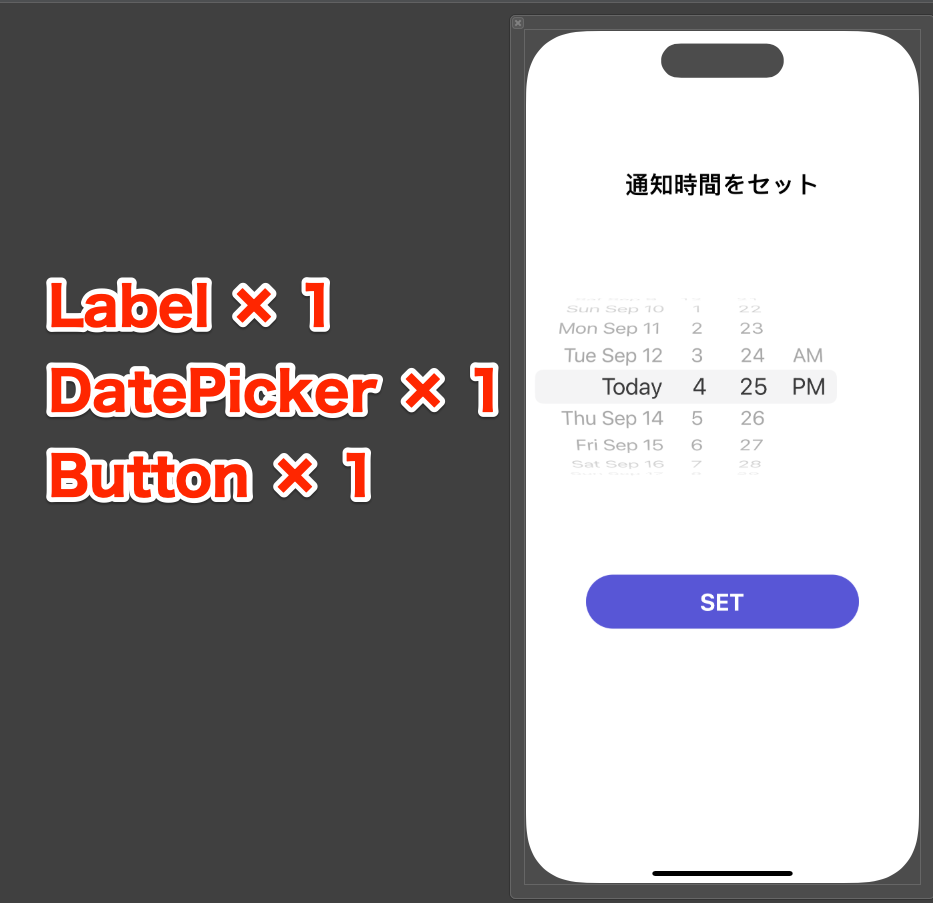
AppDelegate.swift
import UIKit
import UserNotifications
@main
class AppDelegate: UIResponder, UIApplicationDelegate, UNUserNotificationCenterDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
let center = UNUserNotificationCenter.current() // ユーザに通知の許可を求める
center.requestAuthorization(options: [.alert, .sound]) { (granted, error) in
// Enable or disable features based on authorization
}
center.delegate = self
return true
}
// アプリがフォアグラウンドの時に通知を受け取ると呼ばれるメソッド
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
completionHandler([.banner, .list, .sound])
}
// MARK: UISceneSession Lifecycle
func application(_ application: UIApplication, configurationForConnecting connectingSceneSession: UISceneSession, options: UIScene.ConnectionOptions) -> UISceneConfiguration {
// Called when a new scene session is being created.
// Use this method to select a configuration to create the new scene with.
return UISceneConfiguration(name: "Default Configuration", sessionRole: connectingSceneSession.role)
}
func application(_ application: UIApplication, didDiscardSceneSessions sceneSessions: Set<UISceneSession>) {
// Called when the user discards a scene session.
// If any sessions were discarded while the application was not running, this will be called shortly after application:didFinishLaunchingWithOptions.
// Use this method to release any resources that were specific to the discarded scenes, as they will not return.
}
}
HomeViewController.swift (メインのVC)
import UIKit
import UserNotifications
final class HomeViewController: UIViewController {
// MARK: - Properties
@IBOutlet private weak var dateLabel: UILabel!
@IBOutlet private weak var datePicker: UIDatePicker!
@IBOutlet private weak var registerButton: DesignableButton!
// MARK: - View Life Cycle
override func viewDidLoad() {
super.viewDidLoad()
}
@IBAction func registerAction(_ sender: Any) {
let content = UNMutableNotificationContent()
content.title = "通知だよ" // 通知タイトル
content.body = "確認してね" // 通知内容
content.sound = UNNotificationSound.default // 通知音
// ローカル通知が発動するTrigger(日付マッチ)を作成
let calendar = Calendar.current
let dateComponents = calendar.dateComponents([.year, .month, .day, .hour, .minute], from: datePicker.date)
let trigger = UNCalendarNotificationTrigger(dateMatching: dateComponents, repeats: false)
// identifier, content, triggerからローカル通知を作成
let identifier = "MyNotification"
let request = UNNotificationRequest(identifier: identifier, content: content, trigger: trigger)
let center = UNUserNotificationCenter.current()
center.add(request) { (error) in
print(error ?? "ローカル通知登録 OK") // error が nil ならローカル通知の登録に成功したと表示します。errorが存在すればerrorを表示します。
}
// 未通知のローカル通知一覧をログ出力
center.getPendingNotificationRequests { (requests: [UNNotificationRequest]) in
for request in requests {
print("/---------------")
print(request)
print("---------------/")
}
}
guard let hour = dateComponents.hour, let minute = dateComponents.minute else { return }
dateLabel.text = "\(hour)時\(minute)分に通知が届くよ!"
}
}