JSON (JavaScript Object Notation) is a popular data interchange format used in many modern applications, including iOS apps. When working with JSON data in Swift, SwiftyJSON is a powerful and easy-to-use library that can save you a lot of time and effort. In this blog post, we will learn how to use SwiftyJSON in SwiftUI to parse and display JSON data.
Contents 非表示
Setup
Before we start, make sure you have SwiftyJSON installed in your Xcode project. You can either download the library from the official website or use a package manager like CocoaPods or Carthage to install it. Once you have installed SwiftyJSON, you can start using it in your SwiftUI app.
Parsing JSON Data
To parse JSON data using SwiftyJSON, you need to convert the data into a Data
object first. Then, you can use the JSON()
initializer to create a SwiftyJSON object from the data. Here’s an example of how to parse JSON data in SwiftUI:
import SwiftyJSON
import SwiftUI
struct Movie: Identifiable {
let id = UUID()
let title: String
let year: Int
let posterURL: URL?
init(json: JSON) {
title = json["Title"].stringValue
year = json["Year"].intValue
posterURL = URL(string: json["Poster"].stringValue)
}
}
class MovieStore: ObservableObject {
@Published var movies = [Movie]()
init() {
let url = URL(string: "https://www.omdbapi.com/?s=star%20wars&apikey=<#yourAPIKey#>")!
URLSession.shared.dataTask(with: url) { data, response, error in
if let data = data {
let json = JSON(data)
let movieArray = json["Search"].arrayValue
self.movies = movieArray.map { Movie(json: $0) }
}
}.resume()
}
}
This code defines a Movie
struct that represents a single movie object. The MovieStore
class is responsible for downloading the movie data from the OMDB API and parsing it using SwiftyJSON. In the init()
method, we use the dataTask(with:completionHandler:)
method of the URLSession
class to download the JSON data from the API. Then, we create a SwiftyJSON object from the data using the JSON()
initializer. Finally, we use the map(_:)
method to convert the JSON array into an array of Movie
objects.
◎ Get your API key from here
Displaying JSON Data in SwiftUI
To display the parsed JSON data in a SwiftUI view, we can use the List
view to loop over the array of Movie
objects and display each movie’s title and year. Here’s an example of how to display the parsed JSON data in a List
view:
import SwiftUI
struct MovieListView: View {
@ObservedObject var store = MovieStore()
var body: some View {
NavigationView {
List(store.movies) { movie in
HStack() {
VStack(alignment: .leading) {
Text(movie.title)
.font(.headline)
Text("Year: \(String(movie.year))")
.font(.subheadline)
}
Spacer()
movie.posterURL.map {
AsyncImage(url: $0) { phase in
switch phase {
case .empty:
ProgressView()
case .success(let image):
image.resizable()
.frame(width: 60, height: 60)
.cornerRadius(8)
case .failure:
Image(systemName: "xmark.square.fill")
@unknown default:
EmptyView()
}
}
.frame(width: 50, height: 50)
}
}
}
.navigationBarTitle("Movies")
}
}
}
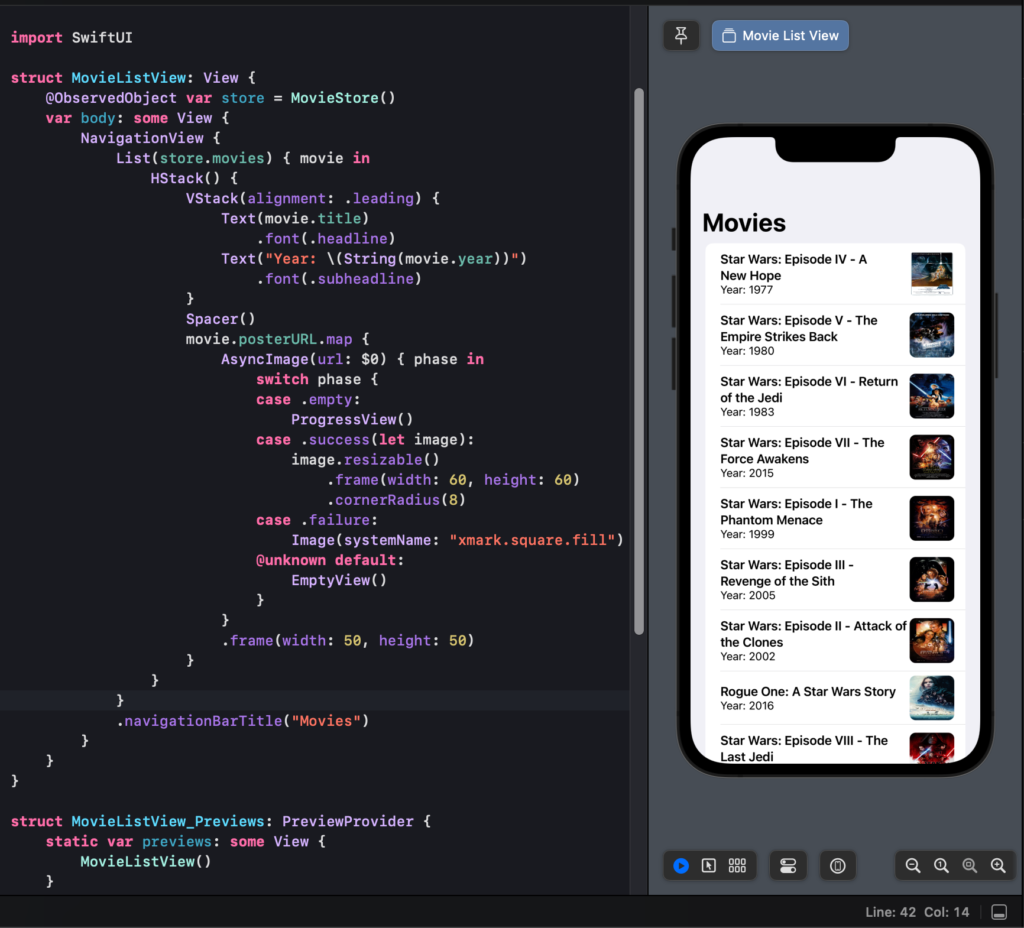
This code defines a MovieListView
struct that displays a list of movies fetched from the OMDB API using the MovieStore
class. We use the List
view to display the array of Movie
objects, and the VStack
view to display each movie’s title and year.
Conclusion
SwiftyJSON is a powerful and easy-to-use library for working with JSON data in Swift. It provides a simple and intuitive API that makes it easy to parse and create JSON objects. With SwiftyJSON, we can easily work with JSON data in our SwiftUI apps and make our apps more dynamic and responsive.