シンプルなEmailとPasswordでログイン or 新規登録ができる画面を作って、エラーが起きた時はエラーメッセージを表示してみましょう。
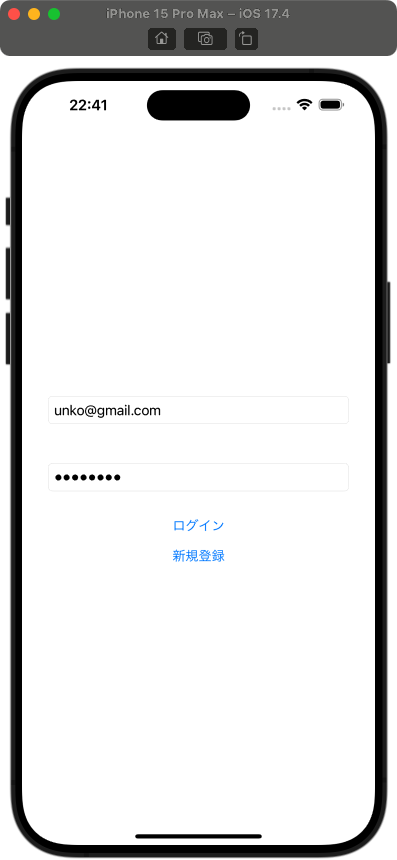
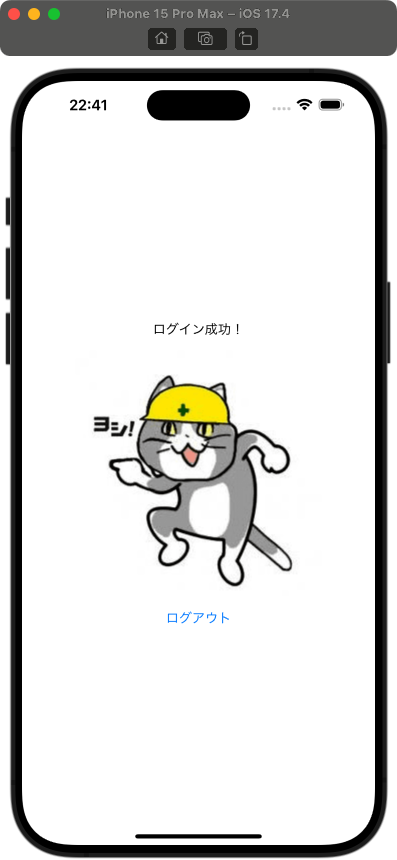

Firebase自体のセットアップは終わっている前提です🙏
FirebaseAuthentication欄のメール/パスワード
だけ有効にしておいてください。
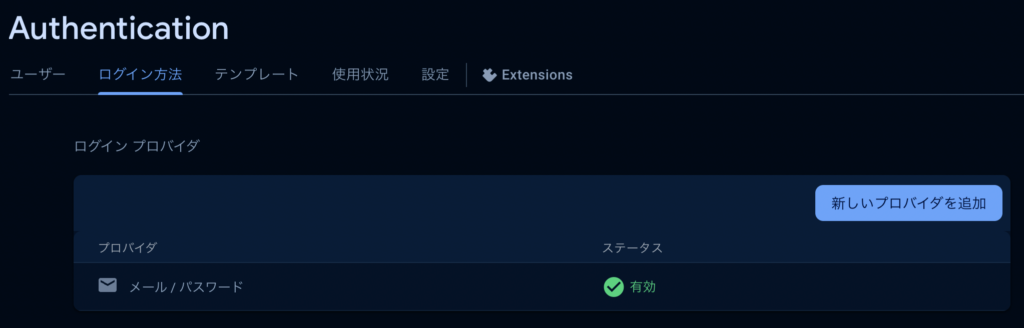
Contents 非表示
実装
HomeView (メインのView)
import SwiftUI
struct HomeView: View {
@State private var email: String = ""
@State private var password: String = ""
@StateObject var authManager = AuthManager()
var body: some View {
VStack(spacing: 16) {
if authManager.isLoggedIn {
Text("ログイン成功!")
Image("img_yoshi") // 画像は個人で好きなものを
.resizable()
.frame(width: 300, height: 300)
Button {
authManager.logout()
} label: {
Text("ログアウト")
}
} else {
TextField("メールアドレス", text: $email)
.textFieldStyle(RoundedBorderTextFieldStyle())
.padding()
SecureField("パスワード", text: $password)
.textFieldStyle(RoundedBorderTextFieldStyle())
.padding()
Button {
authManager.login(email: email, password: password)
} label: {
Text("ログイン")
}
Button {
authManager.register(email: email, password: password)
} label: {
Text("新規登録")
}
}
if let errorMessage = authManager.errorMessage {
Text(errorMessage)
.foregroundColor(.red)
}
}
.padding()
}
}
ログイン / 新規登録 / ログアウト管理用ファイル
ここではわかりやすくAuthManager
にします。
import SwiftUI
import FirebaseAuth
final class AuthManager: ObservableObject {
@Published var errorMessage: String?
@Published var isLoggedIn = false
// ログイン
func login(email: String, password: String) {
Auth.auth().signIn(withEmail: email, password: password) { [weak self] result, error in
if let error = error {
self?.errorMessage = error.localizedDescription
} else {
self?.isLoggedIn = true
self?.errorMessage = nil
}
}
}
// 新規登録
func register(email: String, password: String) {
Auth.auth().createUser(withEmail: email, password: password) { [weak self] result, error in
if let error = error {
self?.errorMessage = error.localizedDescription
} else {
self?.isLoggedIn = true
self?.errorMessage = nil
}
}
}
// ログアウト
func logout() {
do {
try Auth.auth().signOut()
isLoggedIn = false
} catch {
errorMessage = error.localizedDescription
}
}
}
エラーの時はエラーメッセージが表示されます。
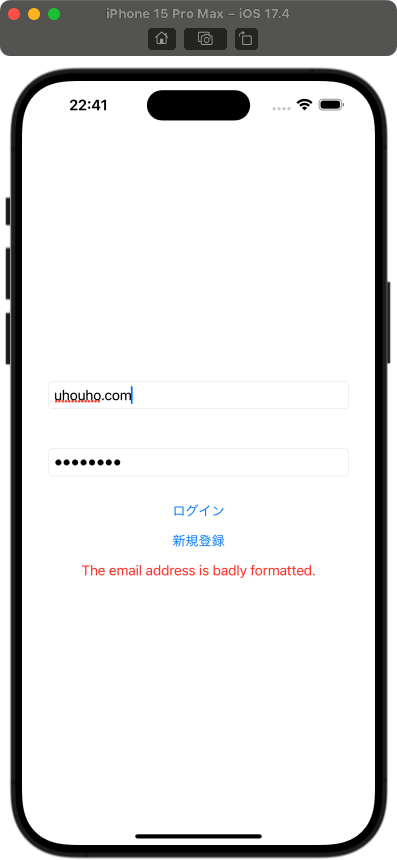
また登録アカウント一覧はFirebaseコンソールで確認ができます。
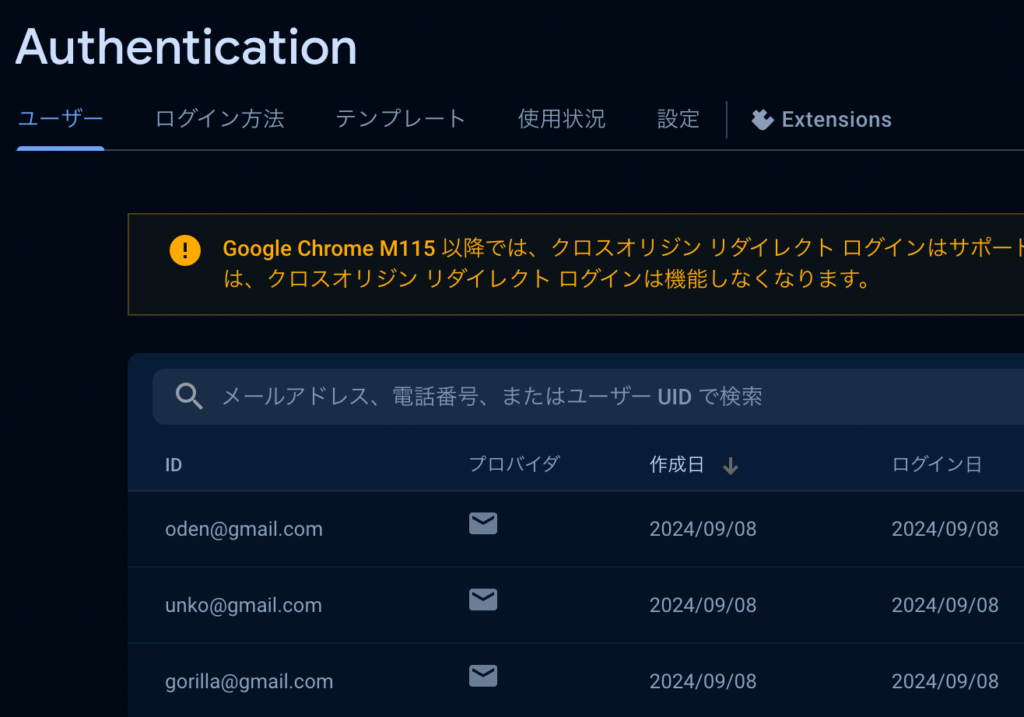