APIs are a critical component of modern mobile development, allowing mobile applications to interact with remote servers and exchange data. One common use case of APIs is fetching data from a remote server and displaying it on a list in a user interface. In this blog article, we will explore how to fetch data from an API and display it on a list in SwiftUI, Apple’s modern UI framework for iOS, macOS, watchOS, and tvOS.
Defining the API endpoint and data model
The first step in fetching data from an API in SwiftUI is defining the API endpoint and data model. We need to define the structure of the data we want to retrieve, as well as the URL of the API endpoint. For example, if we want to fetch a list of users from a remote server, we might define the data model as follows:
struct User: Codable, Identifiable {
let id: Int
let name: String
let email: String
}
And the API endpoint might look like this:
https://jsonplaceholder.typicode.com/users
Fetching data from the API endpoint
Once we have defined the API endpoint and data model, the next step is to fetch the data from the endpoint. In SwiftUI, we can use the URLSession
API to send a GET request to the API endpoint and retrieve the data. Here is an example of how to fetch data from an API endpoint using URLSession
:
class UserViewModel: ObservableObject {
@Published var users = [User]()
func fetchUsers() {
guard let url = URL(string: "https://jsonplaceholder.typicode.com/users") else {
return
}
URLSession.shared.dataTask(with: url) { (data, response, error) in
if let error = error {
print(error.localizedDescription)
} else if let data = data {
do {
let decoder = JSONDecoder()
let users = try decoder.decode([User].self, from: data)
DispatchQueue.main.async {
self.users = users
}
} catch {
print(error.localizedDescription)
}
}
}.resume()
}
}
In this example, we define a UserViewModel
class that conforms to the ObservableObject
protocol. We use the @Published
property wrapper to publish changes to the users
array when it is updated. We define a fetchUsers()
method that sends a GET request to the API endpoint using URLSession
, and parses the response data using JSONDecoder
.
Displaying the data on a list
Now that we have retrieved the data from the API endpoint, the final step is to display it on a list in our user interface. In SwiftUI, we can use the List
view to display the data on a list. Here is an example of how to display the list of users using List
:
struct UserListView: View {
@ObservedObject var viewModel = UserViewModel()
var body: some View {
NavigationView {
List(viewModel.users) { user in
Text(user.name)
}
.navigationBarTitle("Users")
}
.onAppear {
self.viewModel.fetchUsers()
}
}
}
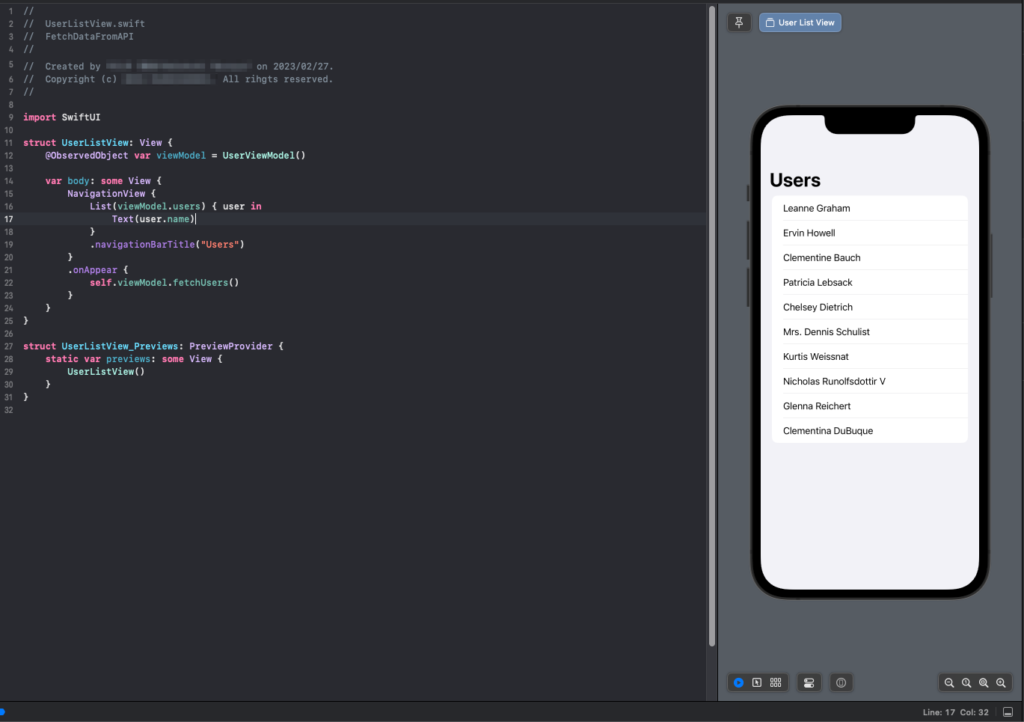
In this example, we define a UserListView
view that displays the list of users using the List
view. We use the @ObservedObject
property wrapper to observe changes to the UserViewModel
instance. We call the fetchUsers()
method in the onAppear
modifier to fetch the data from the API endpoint when the view appears on the screen. The List
view takes an array of users as its data source, and for each user, it displays the user’s name using a Text
view. We also set the title of the navigation bar to “Users” using the navigationBarTitle
modifier.
Conclusion
In this blog article, we explored how to fetch data from an API and display it on a list in SwiftUI. We started by defining the API endpoint and data model, then we fetched the data using URLSession
and parsed the response using JSONDecoder
. Finally, we displayed the list of users using the List
view in our user interface. SwiftUI’s declarative syntax and support for reactive programming with the ObservableObject
protocol make it easy to fetch and display data from an API in our mobile applications.