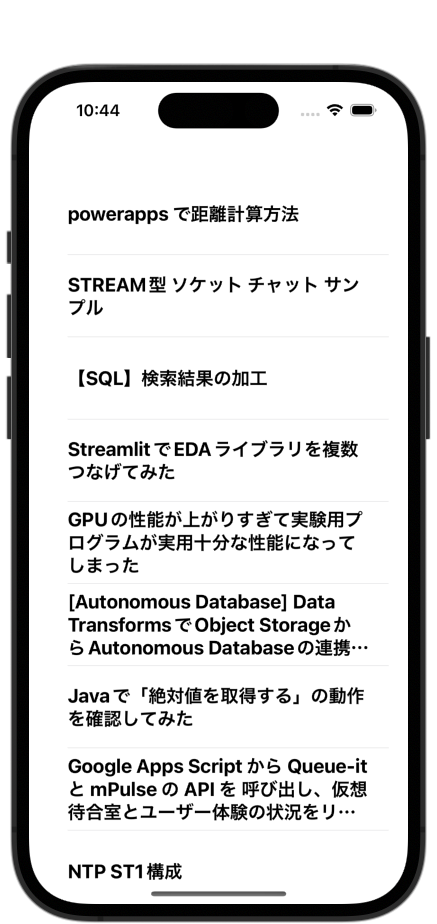
Contents 非表示
実装
Alamofireのインストール
pod 'Alamofire'
HomeViewController.xib (メインのVC、StoryboardでもOK)
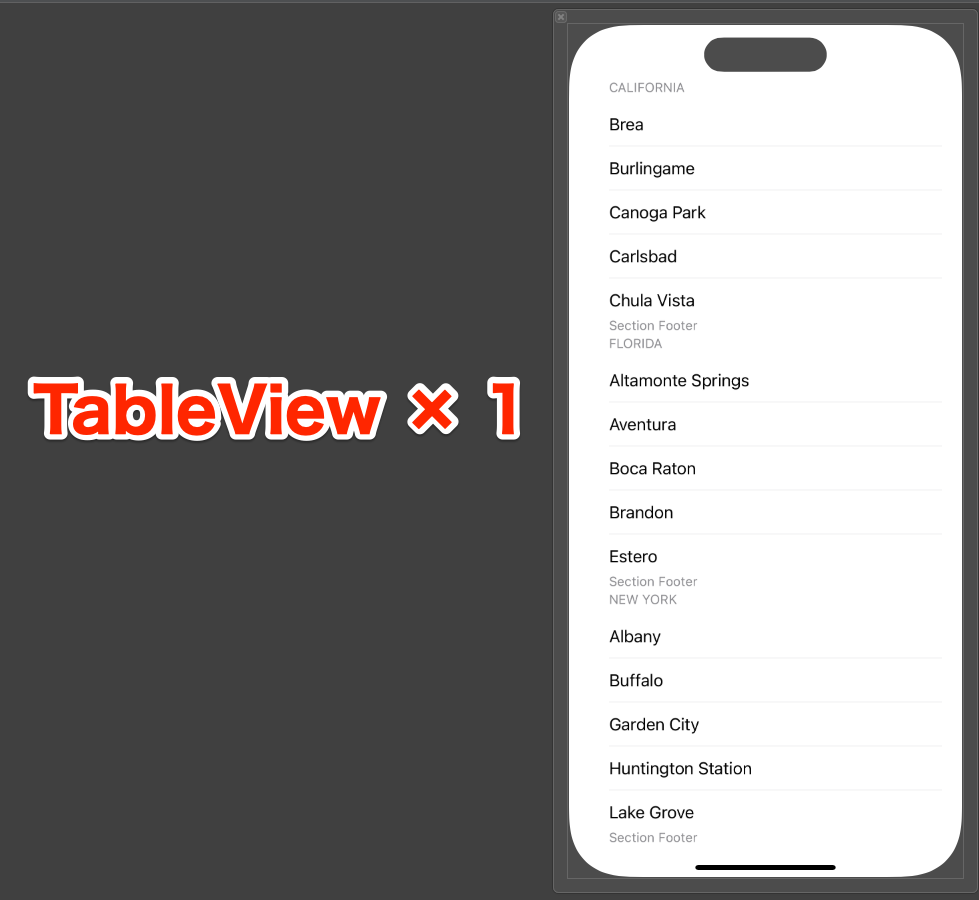
HomeTableViewCell.xib
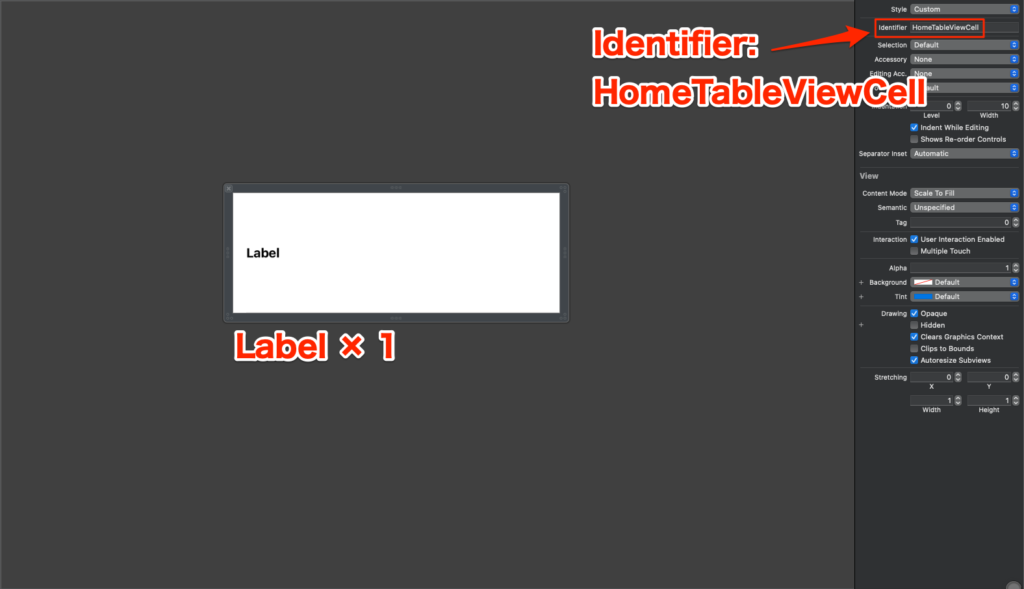
Article.swift (Model)
import Foundation
struct Article: Codable {
let title: String
init(title: String) {
self.title = title
}
}
HomeTableViewCell.swift
import UIKit
class HomeTableViewCell: UITableViewCell {
@IBOutlet private weak var articleLabel: UILabel!
override func prepareForReuse() {
super.prepareForReuse()
articleLabel.text = nil
}
func configure(_ article: String) {
articleLabel.text = article
}
}
HomeViewController.swift
import UIKit
import Alamofire
final class HomeViewController: UIViewController {
// MARK: - Properties
@IBOutlet private weak var tableView: UITableView!
private let decoder: JSONDecoder = JSONDecoder()
private var article: [Article] = []
// MARK: - View Life Cycle
override func viewDidLoad() {
super.viewDidLoad()
setupTableView()
getQiitaArticles()
}
private func setupTableView() {
tableView.delegate = self
tableView.dataSource = self
tableView.register(UINib(nibName: "HomeTableViewCell", bundle: nil), forCellReuseIdentifier: "HomeTableViewCell")
}
private func getQiitaArticles() {
AF.request("https://qiita.com/api/v2/items").responseDecodable(of: [Article].self) { response in
switch response.result {
case .success(let articles):
self.article = articles
self.tableView.reloadData()
case .failure(let error):
print("Error: \(error)")
}
}
}
}
// MARK: - TableView
extension HomeViewController: UITableViewDelegate, UITableViewDataSource {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return article.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
guard let cell = tableView.dequeueReusableCell(withIdentifier: "HomeTableViewCell", for: indexPath) as? HomeTableViewCell else {
return UITableViewCell()
}
cell.configure(article[indexPath.row].title)
return cell
}
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return self.view.frame.height / 10
}
}