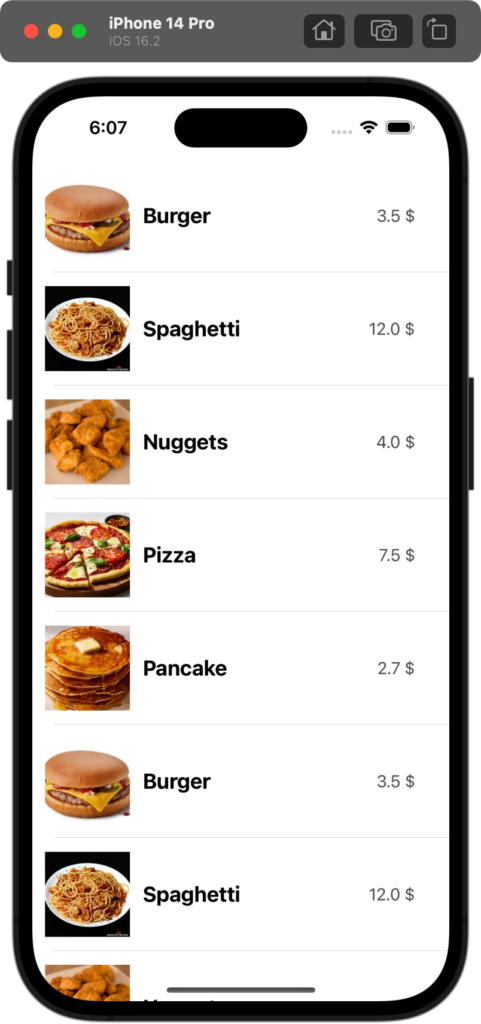
Contents 非表示
実装
View側
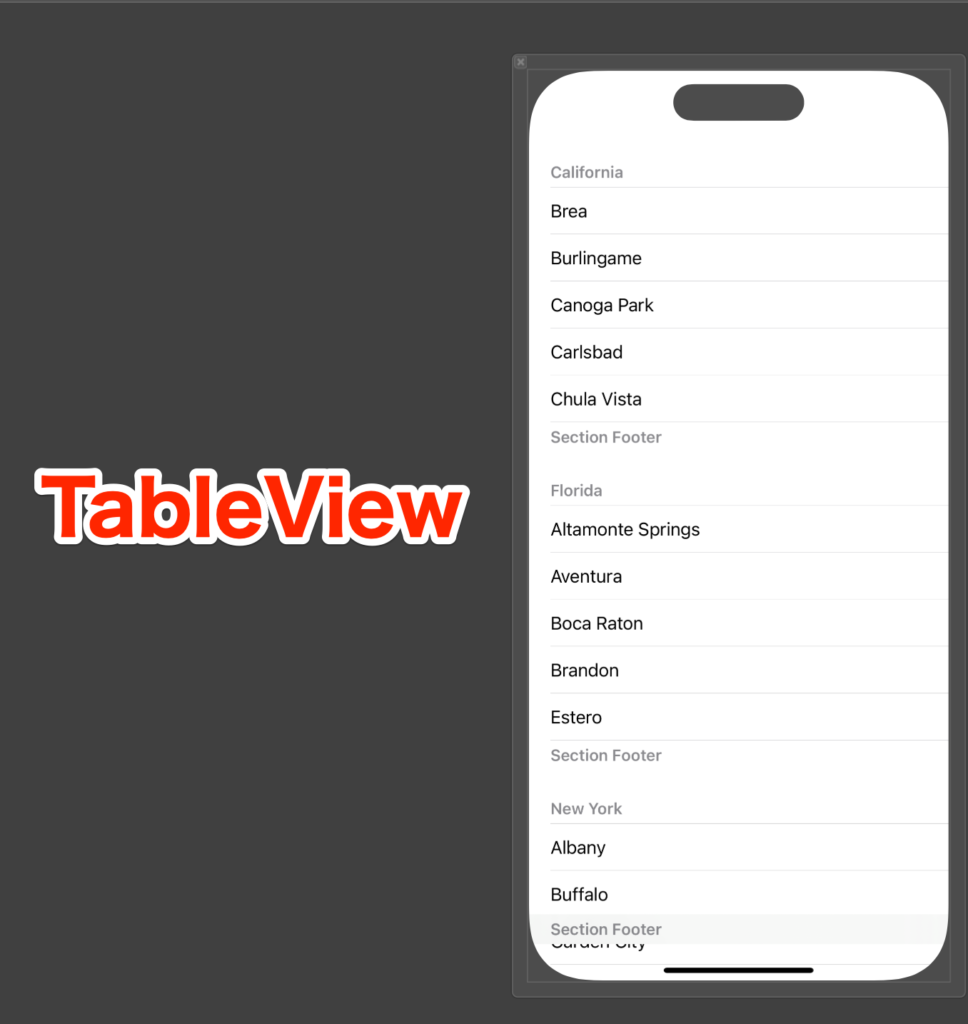
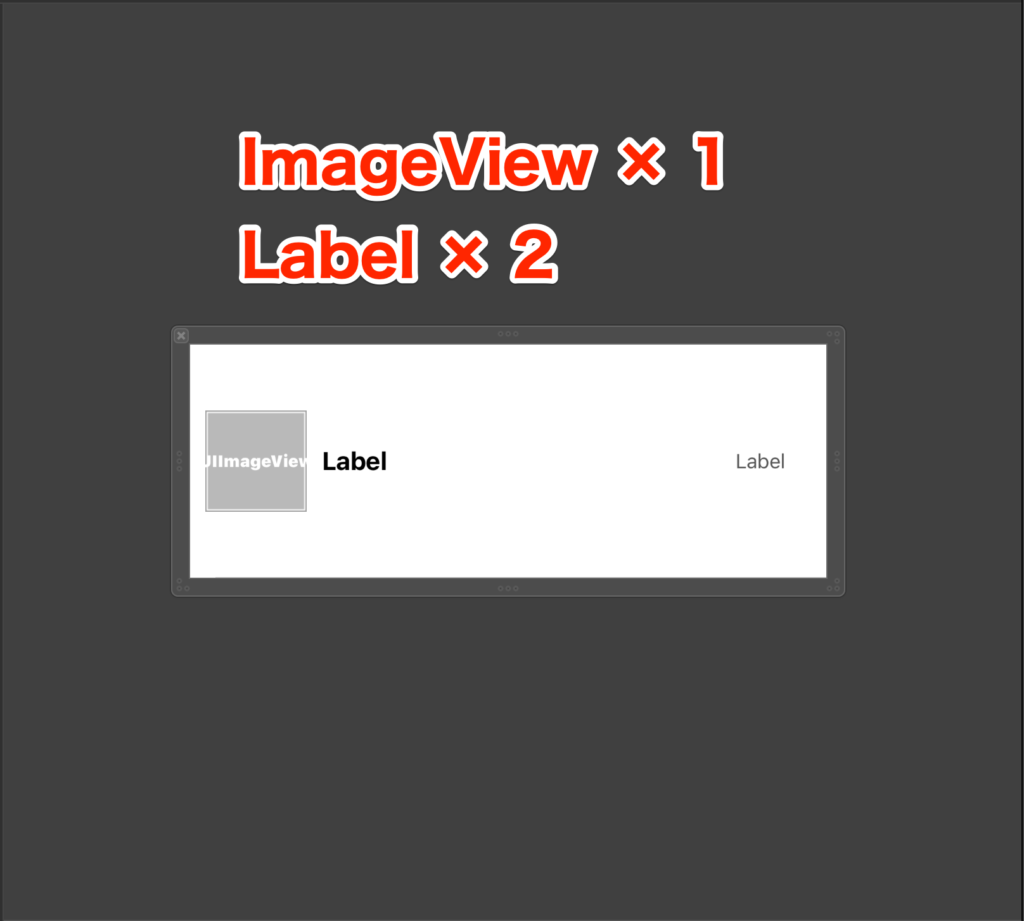
コード側 (ViewController)
import UIKit
final class HomeViewController: UIViewController {
// MARK: - Properties
@IBOutlet private weak var tableView: UITableView!
// タプルの配列
private let menu: [(image: UIImage, name: String, price: Double)] = [
(Asset.Assets.burger.image, "Burger", 3.5), (Asset.Assets.spaghetti.image, "Spaghetti", 12.0), (Asset.Assets.nuggets.image, "Nuggets", 4.0), (Asset.Assets.pizza.image, "Pizza", 7.5), (Asset.Assets.pancakes.image, "Pancake", 2.7), (Asset.Assets.burger.image, "Burger", 3.5), (Asset.Assets.spaghetti.image, "Spaghetti", 12.0), (Asset.Assets.nuggets.image, "Nuggets", 4.0), (Asset.Assets.pizza.image, "Pizza", 7.5), (Asset.Assets.pancakes.image, "Pancake", 2.7)
]
// MARK: - View Life Cycle
override func viewDidLoad() {
super.viewDidLoad()
setupTableView()
}
private func setupTableView() {
tableView.delegate = self
tableView.dataSource = self
tableView.register(UINib(nibName: "HomeTableViewCell", bundle: nil), forCellReuseIdentifier: "HomeTableViewCell")
}
}
// MARK: - TableView
extension HomeViewController: UITableViewDelegate, UITableViewDataSource {
// セクション内のRowの数
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return menu.count
}
// セルに表示させたいものの設定
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
guard let cell = tableView.dequeueReusableCell(withIdentifier: "HomeTableViewCell", for: indexPath) as? HomeTableViewCell else {
return UITableViewCell()
}
cell.configure(image: menu[indexPath.row].image, name: menu[indexPath.row].name, price: menu[indexPath.row].price)
return cell
}
// 高さ
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return self.view.frame.height / 8
}
}
import UIKit
final class HomeTableViewCell: UITableViewCell {
// MARK: - Properties
@IBOutlet private weak var menuImageView: UIImageView!
@IBOutlet private weak var menuNameLabel: UILabel!
@IBOutlet private weak var menuPriceLabel: UILabel!
// MARK: - Initialize
override func prepareForReuse() {
super.prepareForReuse()
menuImageView.image = nil
menuNameLabel.text = nil
menuPriceLabel.text = nil
}
func configure(image: UIImage, name: String, price: Double) {
menuImageView.image = image
menuNameLabel.text = name
menuPriceLabel.text = "\(String(price)) $"
}
}