今回の内容
・変数とか関数のテンプレを備忘録として残してみる
Swiftを勉強し始めた初日の自分に向けてテンプレを残してみました、型の明記は不要だったり引数が複数あったりすることもありますが、全パターンは書ききれないので基本的な記述方式だけ残しています。(余裕があれば随時追記していきたい)
Contents 非表示
テンプレ一覧 (UIKit, Storyboard)
定数
private let <#定数名#>: <#型#> = <#値#>
// 例
private let number: Int = 0
変数
private var <#定数名#>: <#型#> = <#値#>
// 例
private var nameString: String = ""
配列
private let <#名前#>: [<#配列要素の型#>] = []
// 例
private let nameArray: [String] = ["Taro", "Jiro", "Saburo"]
タプル
private var <#変数名#>: (<#引数名1#>: <#型#>, <#引数名2#>: <#型#>) = (<#引数名1#>: <#値#>, <#引数名2#>: <#値#>)
// 例
private var personData: (name: String, age: Int) = (name: "", age: 0)
関数
// 引数 & 戻り値なし
private func <#関数名#>() {
<#処理#>
}
// 引数あり
private func <#関数名#>(<#引数名#>: <#型#>) {
<#処理#>
}
// 戻り値あり
private func <#関数名#>() -> <#戻り値の型#> {
<#処理#>
return <#戻り値#>
}
// 引数 & 戻り値あり
private func <#関数名#>(<#引数名#>: <#型#>) -> <#戻り値の型#> {
<#処理#>
return <#戻り値#>
}
// Generics(ジェネリクス)を使用する場合
private func <#関数名#><T>(<#引数名1#>: T, <#引数名2#>: T) -> (T, T) { // 何の型でもOK
return (<#引数名1#>, <#引数名2#>)
}
// 引数 & 戻り値なし (例)
private func simpleFunction() {
print("Hello World!")
}
// 引数あり (例)
private func greeting(name: String) {
print("My name is \(name)")
}
// 戻り値あり (例)
private func calculation() -> Int {
let calculationResult = 10 * 10
return calculationResult
}
// 引数 & 戻り値あり (例)
private func calculateTriangle(baseLength: Int) -> Int {
let triangleArea = baseLength * 10 / 2
return triangleArea
}
// Generics(ジェネリクス)を使用する場合 (例)
private func helloGenerics<T>(first: T, second: T) -> (T, T) {
return (first, second)
}
if let構文
if let <#名前#> = <#値が入っているか確かめたいもの#> {
<#処理#>
}
// 例
let helloString: String = "Hello Japan!"
if let japan = helloString {
print(japan)
}
guard let構文
guard let <#名前#> = <#値が入っているか確かめたいもの#> else { return }
guard let <#名前#> = <#値が入っているか確かめたいもの#> else { fatalError("<#エラー時に出したいログ#>") }
// 例
let myAge: Int = 99
guard let age = myAge else { return }
// FatalErrorを使用する場合
guard let age = myAge else { fatalError("値がないよ") }
幅&高さ指定
self.view.frame.<#WidthorHeight#> <#*とか/#> <#数値#>
// 例
self.view.frame.width / 6 // 幅
self.view.frame.height / 6 // 高さ
StoryboardIDを使った画面遷移 (モーダル)
let storyboard = UIStoryboard(name: "<#Storyboard名#>", bundle: nil)
guard let <#名前#>ViewController = storyboard.instantiateViewController(withIdentifier: "<#Identifier#>") as? <#ファイル名#>ViewController else { return }
self.present(<#名前#>ViewController, animated: true)
// 例
let storyboard = UIStoryboard(name: "Next", bundle: nil)
guard let nextViewController = storyboard.instantiateViewController(withIdentifier: "NextViewController") as? NextViewController else { return }
// nextViewController.modalPresentationStyle = .fullScreen // フルスクリーンモーダルにしたい場合
self.present(nextViewController, animated: true)
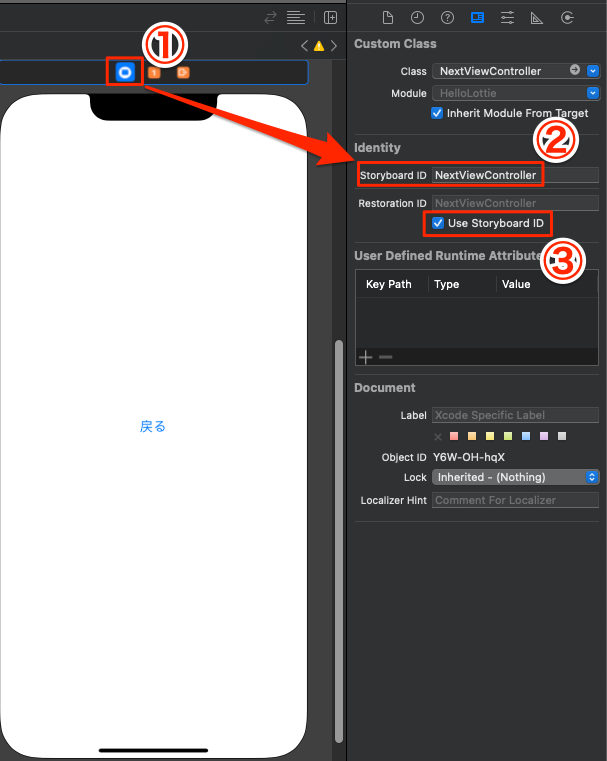
Xib開発の画面遷移 (モーダル)
let <#名前#>ViewController = <#ファイル名#>ViewController(nibName: "<#名前#>", bundle: nil)
present(<#名前#>ViewController, animated: true)
// 例
let nextViewController = NextViewController(nibName: "NextViewController", bundle: nil)
// nextViewController.modalPresentationStyle = .fullScreen // フルスクリーンモーダルにしたい場合
present(nextViewController, animated: true)
Xib開発の画面遷移 (ナビゲーション)
◎SceneDelegate.swift
func scene(_ scene: UIScene, willConnectTo session: UISceneSession, options connectionOptions: UIScene.ConnectionOptions) {
guard let _ = (scene as? UIWindowScene) else { return }
// 追記 -----ここから
window = UIWindow(windowScene: scene as! UIWindowScene)
window?.makeKeyAndVisible()
let <#名前#>ViewController = <#ファイル名#>ViewController()
let rootViewController = UINavigationController(rootViewController: <#名前#>ViewController)
window?.rootViewController = rootViewController
// 追記 -----ここまで
}
let <#名前#>ViewController = <#遷移先の#>ViewController()
self.navigationController?.pushViewController(<#名前#>ViewController, animated: true)
// 例
let nextViewController = NextViewController()
self.navigationController?.pushViewController(nextViewController, animated: true)
テンプレ一覧 (SwiftUI)
@State
@State private var <#変数名#>: <#型#> = <#値#>
// 例
@State private var number: Int = 0
@Binding
@Binding var <#変数名#>: <#型#>
// バインディングしたいもの
<#名前#>View(<#バインディング変数#>: $<#@State変数とか#>)
// 例
@Binding var number: Int
// バインディングしたいもの
NextView(number: $number)
ForEach
ForEach(<#回したい回数#>, id: \.self) { index in
<#ButtonとかImage#>
}
// 例
ForEach(0..<5, id: \.self) { index in
Button {
print("Index: \(index)")
} label: {
Text("Hello\(index)")
}
}
List
@State private var <#配列名#> = [<#要素#>]
List(<#配列名#>, id: \.self) { index in
Text(index)
}
// 例
@State private var fruitsArray = ["Apple", "Banana", "Mango", "Grapes"]
List(fruitsArray, id: \.self) { fruitIndex in
Text(fruitIndex)
}
TextField
@State private var <#変数名#> = ""
TextField("<#Placeholder#>", text: self.$<#変数名#>)
// 例
@State private var name = ""
TextField("名前を入力", text: self.$name)
Modal遷移
@State private var <#変数名#> = false
Button {
<#変数名#> = true
} label: {
Text("NextViewへ")
}
.<#sheet or fullScreenCover#>(isPresented: $<#変数名#>) {
<#遷移先の#>View()
}
// 例
@State private var isShowNextView = false
Button {
isShowNextView = true
} label: {
Text("NextViewへ")
}
.sheet(isPresented: $isShowNextView) {
NextView()
}
// // フルスクリーンにしたい場合
// .fullScreenCover(isPresented: $isShowNextView) {
// NextView()
// }
Navigation遷移
@State private var <#変数名#> = false
NavigationStack() {
<#ボタンとかテキスト#>
}
.navigationDestination(isPresented: $<#変数名#>) {
<#遷移先の#>View()
}
@State private var isShowNextView = false
NavigationStack() {
Button {
isShowNextView = true
} label: {
Text("ボタンだよ")
}
}
.navigationDestination(isPresented: $isShowNextView) {
NextView()
}
カスタムモディファイア
import SwiftUI
struct <#Struct名#>: ViewModifier {
let <#定数名#>: <#型#> // 引数にしたいものがある場合
func body(content: Content) -> some View { // カスタムモディファイアの内容
content
<#モディファイア#>
}
}
// 使う時
Text("Hello SwiftUI!")
.modifier(<#Struct名#>(<#引数名(あれば)#>: <#指定するもの#>)
import SwiftUI
// 例
struct ButtonModifier: ViewModifier {
let backgroundColor: Color
func body(content: Content) -> some View {
content
.font(.largeTitle)
.frame(width: UIScreen.main.bounds.width/1.3, height: UIScreen.main.bounds.height/28, alignment: .center)
.padding()
.border(Color.black, width: 1.5)
.foregroundColor(foregroundColor)
.background(backgroundColor)
.padding()
}
}
// 使う時
Text("Hello SwiftUI!")
.modifier(ButtonModifier(backgroundColor: .orange)
幅&高さ指定
UIScreen.main.bounds.<#WidthorHeight#> <#*とか/#> <#数値#>
// 例
UIScreen.main.bounds.width / 2 // 幅
UIScreen.main.bounds.height / 2 // 高さ
ColorのExtension (結構便利)
import SwiftUI
extension Color {
static let <#定数名#> = Color("<#Color Setで設定した名前#>")
}
// 使う時
.foregroundColor(Color.<#定数名#>)
// 例
import SwiftUI
extension Color {
static let mainColor = Color("Main_Color")
}
// 使う時
Button {
print("ボタンが押されたよ")
} label: {
Text("ボタンだよ")
.foregroundColor(Color.mainColor) // こんな感じで使える
}
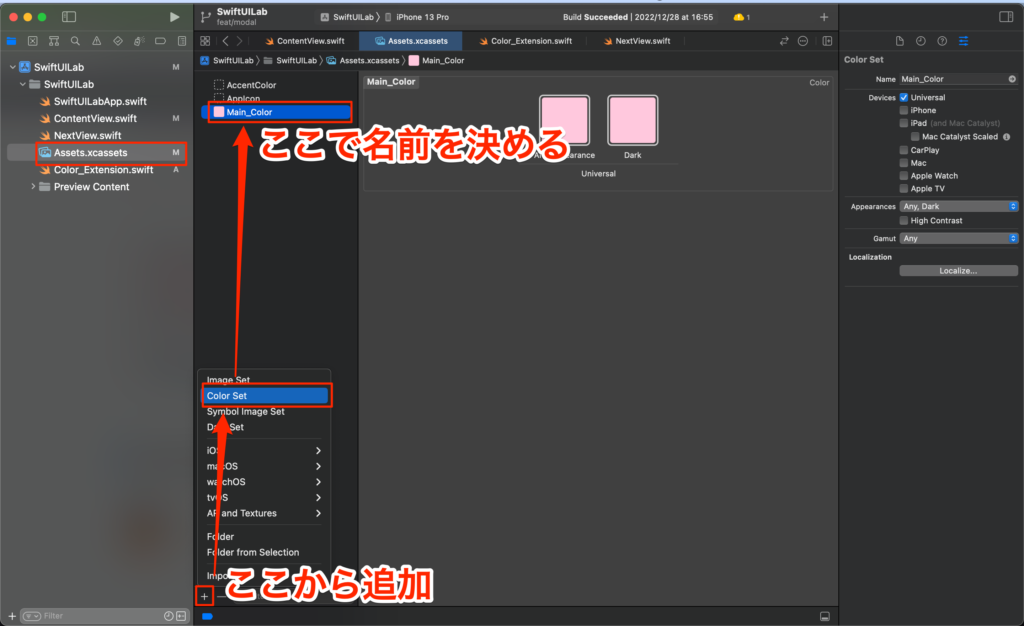
@ViewBuilder
// 引数なし
@ViewBuilder
func <#関数名#>() -> some View {
<#テキストとかボタン#>
.<#モディファイア#>
}
// 引数あり
@ViewBuilder
func <#関数名#>(<#引数名#>: <#型#>) -> some View {
<#テキストとかボタン#>
.<#モディファイア#>
}
// 例
VStack {
ForEach(1..<6, id: \.self) { index in
sampleView(index: index)
}
}
@ViewBuilder
func sampleView(index: Int) -> some View {
Text("Text\(index)")
.padding()
.font(.largeTitle)
}
まとめ(るほどでもない)
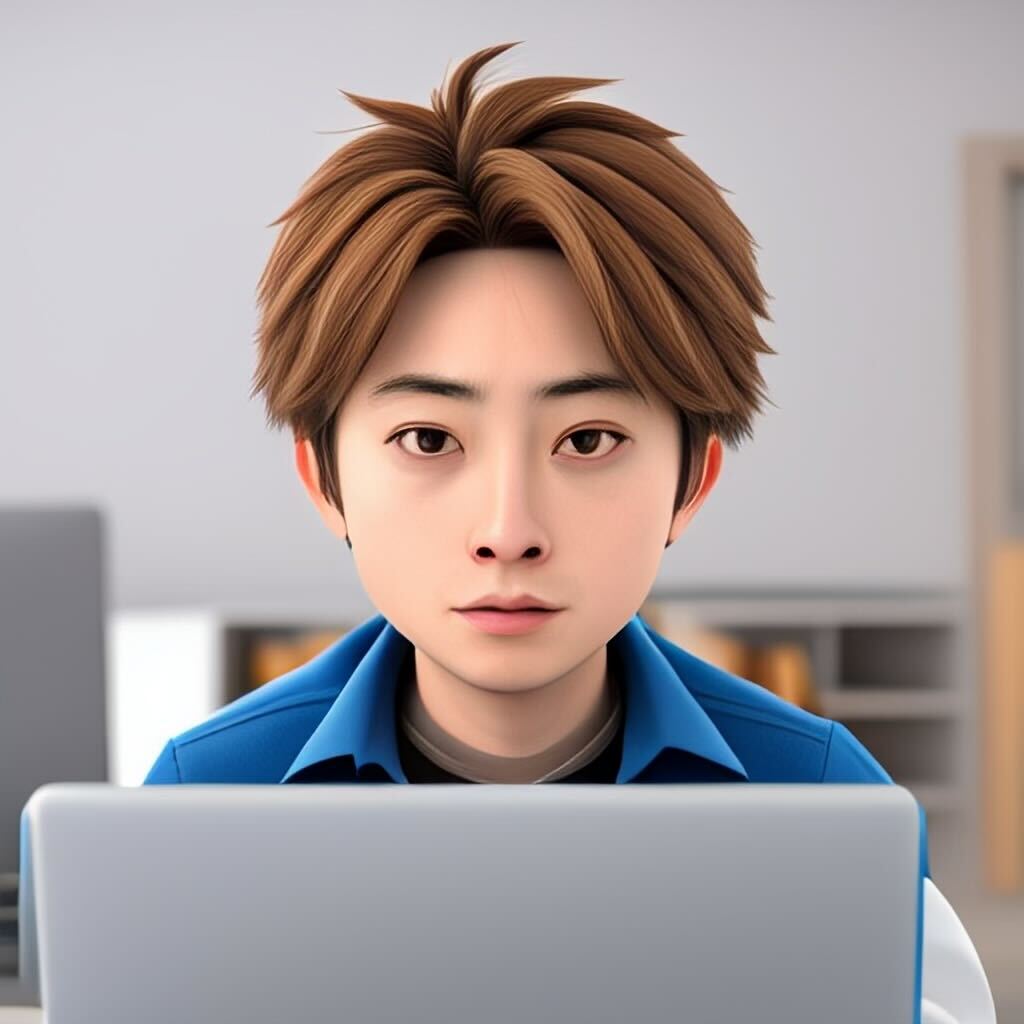
備忘録ってやっぱり大事だなぁ