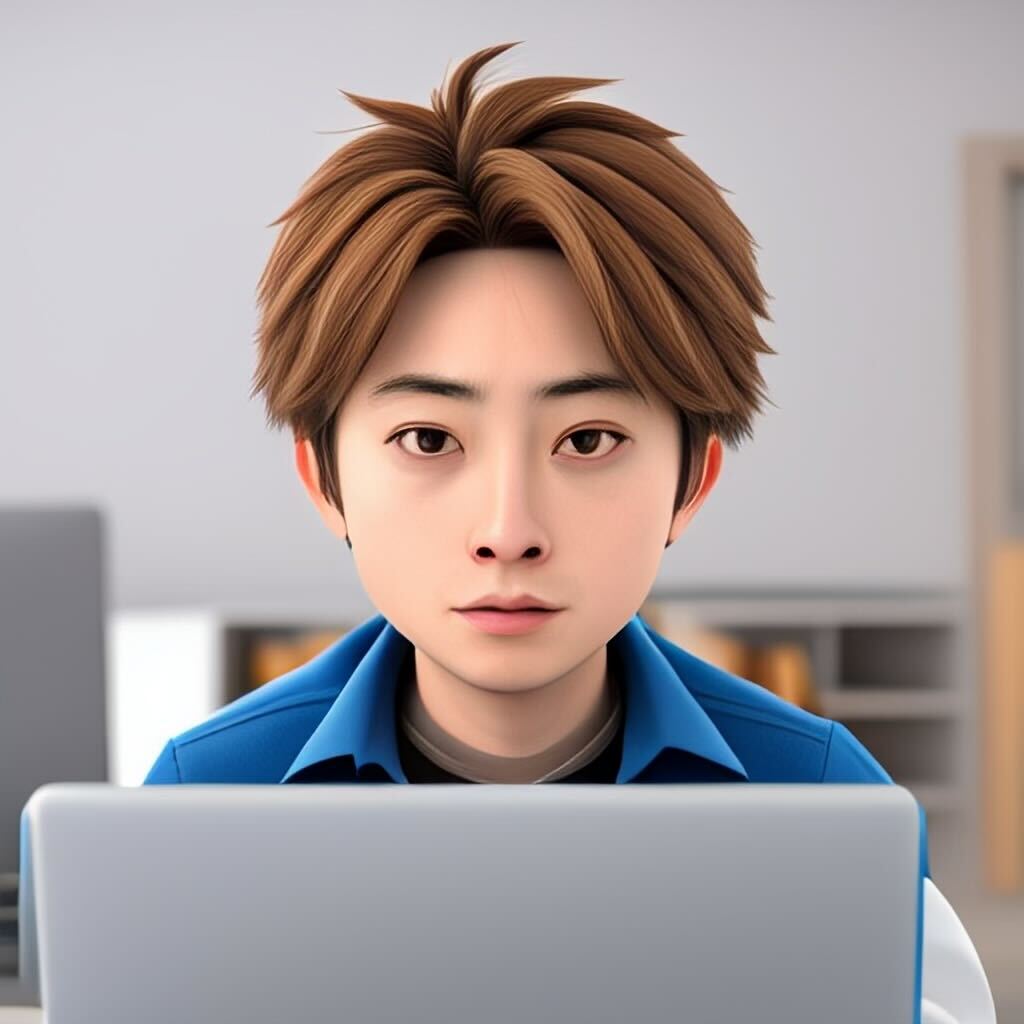
そのままコピペすれば使えます
TableView
import UIKit
class ViewController: UIViewController {
// MARK: - Outlets
@IBOutlet private weak var tableView: UITableView!
// MARK: - View Lifecycle
override func viewDidLoad() {
super.viewDidLoad()
setupTableView()
}
// MARK: - Private Methods
private func setupTableView() {
tableView.delegate = self
tableView.dataSource = self
tableView.register(UINib(nibName: "<#ファイル名#>", bundle: nil), forCellReuseIdentifier: "<#CellのIdentifier#>")
}
}
// MARK: - Extensions
extension <#名前#>ViewController: UITableViewDelegate, UITableViewDataSource {
// セクション数
func numberOfSections(in tableView: UITableView) -> Int {
return <#返したい数#>
}
// セクション内のRowの数
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return <#返したい数#>
}
// セルに表示させたいものの設定
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
guard let cell = tableView.dequeueReusableCell(withIdentifier: "<#CellのIdentifier#>", for: indexPath) as? <#ファイル名#> else {
return UITableViewCell()
}
return cell
}
// 高さ
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return self.view.frame.height / <#分割したい数#>
}
}
import UIKit
class ViewController: UIViewController {
// MARK: - Properties
private let fruitsArray = ["Banana", "Apple", "Orange", "Grape", "Mango", "Strawberry"]
// MARK: - Outlets
@IBOutlet private weak var tableView: UITableView!
// MARK: - View Lifecycle
override func viewDidLoad() {
super.viewDidLoad()
setupTableView()
}
// MARK: - Private Methods
private func setupTableView() {
tableView.delegate = self
tableView.dataSource = self
tableView.register(UINib(nibName: "CustomTableViewCell", bundle: nil), forCellReuseIdentifier: "CustomTableViewCell")
}
}
// MARK: - Extensions
extension ViewController: UITableViewDelegate, UITableViewDataSource {
// セクション数
func numberOfSections(in tableView: UITableView) -> Int {
return fruitsArray.count
}
// セクション内のRowの数
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 6
}
// セルに表示させたいものの設定
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
guard let cell = tableView.dequeueReusableCell(withIdentifier: "CustomTableViewCell", for: indexPath) as? CustomTableViewCell else {
return UITableViewCell()
}
cell.setupCell(fruitsArray[indexPath.row]) // CustomCellファイル内の関数
return cell
}
// 高さ
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return self.view.frame.height / 6
}
}
CollectionView
// MARK: - View Lifecycle
override func viewDidLoad() {
super.viewDidLoad()
setupCollectionView()
}
// MARK: - Private Methods
private func setupCollectionView() {
collectionView.delegate = self
collectionView.dataSource = self
collectionView.register(UINib(nibName: "<#CollectionViewFileName#>", bundle: nil), forCellWithReuseIdentifier: "<#CollectionViewCellIdentifier#>")
}
}
// MARK: - Extensions
extension <#Name#>ViewController: UICollectionViewDataSource {
// セクション数
func numberOfSections(in collectionView: UICollectionView) -> Int {
return <#返したい数#>
}
// セル数
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return <#返したい数#>
}
// セルに値をセット
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
// widthReuseIdentifierにはStoryboardで設定したセルのIDを指定
guard let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "<#CollectionViewCellIdentifier#>", for: indexPath) as? <#CollectionViewFileName#> else {
return UICollectionViewCell()
}
// セルに枠線をセット
cell.layer.borderColor = UIColor.<#つけたい色#>.cgColor // 外枠の色
cell.layer.borderWidth = <#太さ#> // 枠線の太さ
return cell
}
}
// セルのサイズを調整する
extension <#Name#>ViewController: UICollectionViewDelegateFlowLayout {
// セルサイズを指定する
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
// 横方向のサイズを調整
let cellSizeWidth:CGFloat = self.view.frame.width / <#分割したい数#>
let cellSizeHeight:CGFloat = self.view.frame.height / <#分割したい数#>
// widthとheightのサイズを返す
return CGSize(width: cellSizeWidth, height: cellSizeHeight / <#分割したい数#>)
}
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, minimumLineSpacingForSectionAt section: Int) -> CGFloat {
return <#行間#> // 行間
}
}