
今回はスライド形式のチュートリアル画面(のようなもの)を実装します
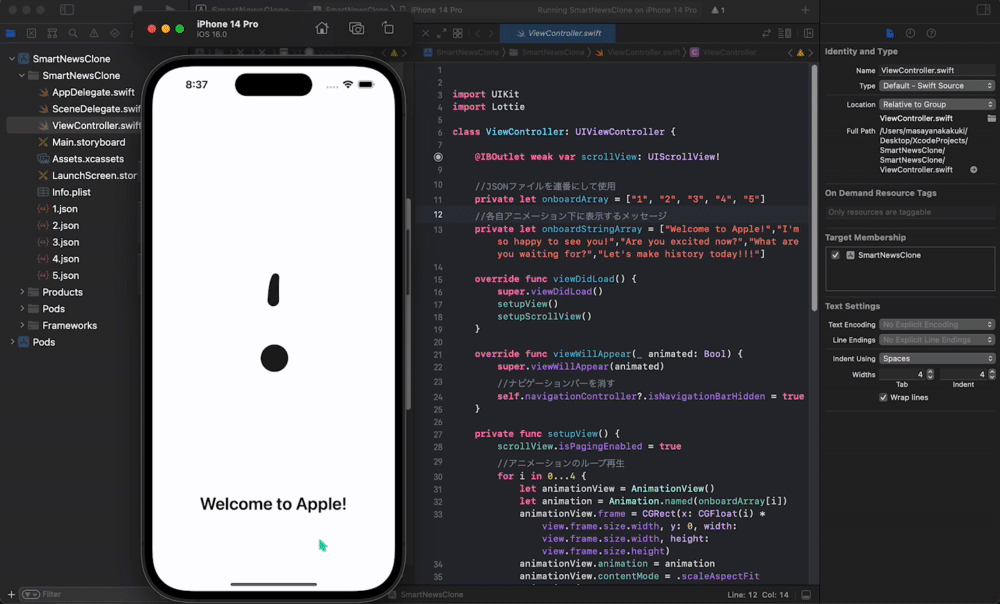
Contents 非表示
開発環境
今回は以下の環境で実装しています。
MacOS | 12.6 |
Xcode | 14.0.1 |
Swift | 5.7 |
Storyboardの装飾 & ライブラリの導入
Storyboard
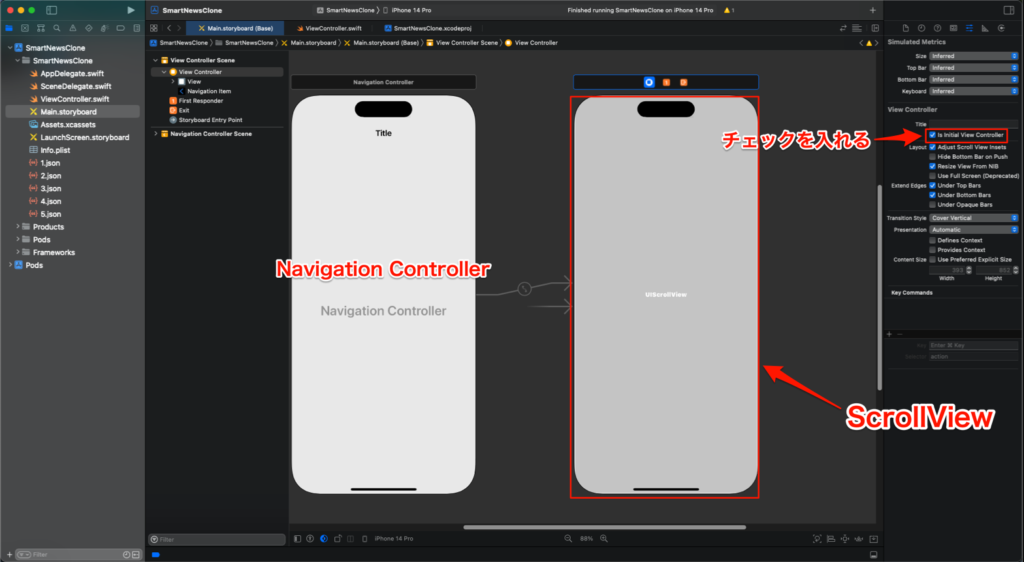
ライブラリの導入
今回はLottieを使用します。
pod 'lottie-ios'
プロジェクトに5つアニメーションを選んでjsonでダウンロード、名前を連番にする。
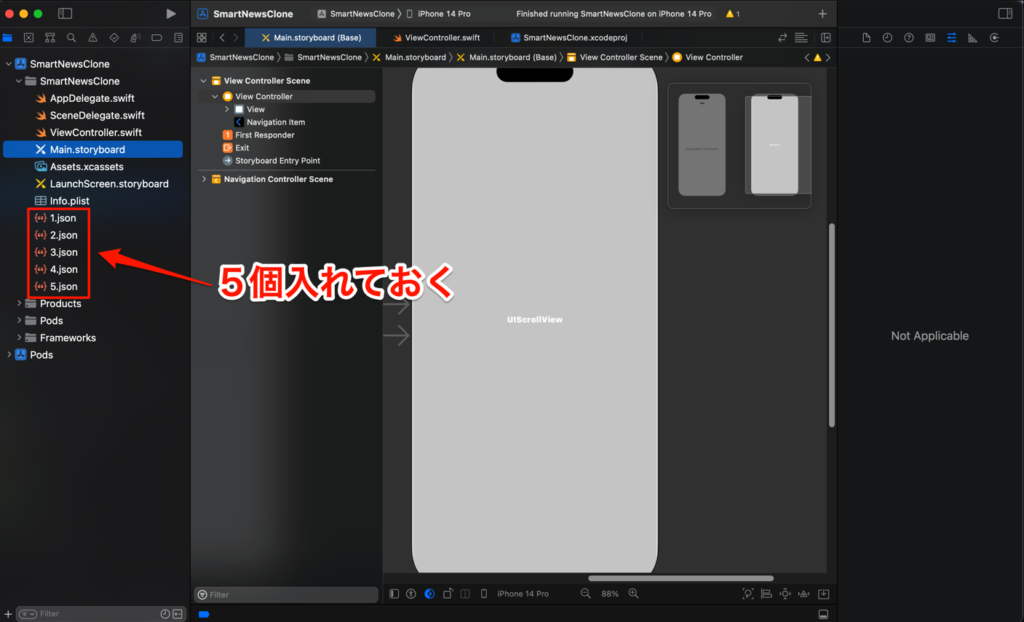
コードの記述
コードの記述
import UIKit
import Lottie
class ViewController: UIViewController {
@IBOutlet weak var scrollView: UIScrollView!
//JSONファイルを連番にして使用
private let onboardArray = ["1", "2", "3", "4", "5"]
//各自アニメーション下に表示するメッセージ
private let onboardStringArray = ["Welcome to Apple!","I'm so happy to see you!","Are you excited now?","What are you waiting for?","Let's make history today!!!"]
override func viewDidLoad() {
super.viewDidLoad()
setupView()
setupScrollView()
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
//ナビゲーションバーを消す
self.navigationController?.isNavigationBarHidden = true
}
private func setupView() {
scrollView.isPagingEnabled = true
//アニメーションのループ再生
for i in 0...4 {
let animationView = AnimationView()
let animation = Animation.named(onboardArray[i])
animationView.frame = CGRect(x: CGFloat(i) * view.frame.size.width, y: 0, width: view.frame.size.width, height: view.frame.size.height)
animationView.animation = animation
animationView.contentMode = .scaleAspectFit
animationView.loopMode = .loop
animationView.play()
scrollView.addSubview(animationView)
}
}
}
extension ViewController: UIScrollViewDelegate {
private func setupScrollView() {
scrollView.delegate = self
scrollView.contentSize = CGSize(width: view.frame.size.width * 5, height: scrollView.frame.size.height)
//表示テキストの設定
for i in 0...4 {
let onboardLabel = UILabel(frame: CGRect(x: CGFloat(i) * view.frame.size.width, y: view.frame.size.height / 3, width: scrollView.frame.size.width, height: scrollView.frame.size.height))
onboardLabel.font = UIFont.boldSystemFont(ofSize: 28.0)
onboardLabel.textAlignment = .center
onboardLabel.text = onboardStringArray[i]
scrollView.addSubview(onboardLabel)
}
}
}
まとめ

今回は以上です、Lottieはこのような場面で大活躍出来るので便利ですね
☆今日の格言
ー Vincent Van Gogh
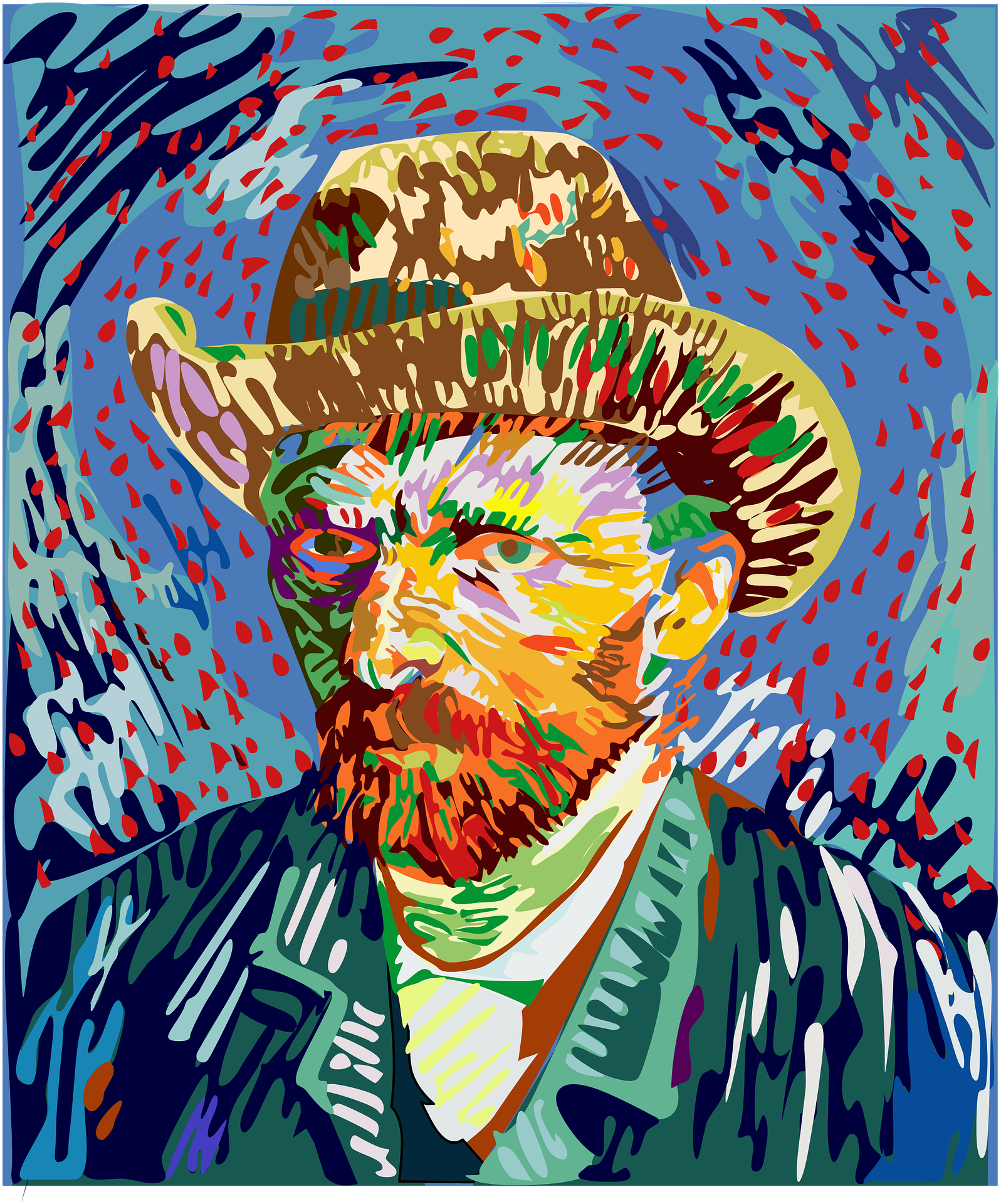
Vincent
There is nothing more truly artistic than to love people.
(人を愛するということ以上に芸術的なものはない)